有一个Student类,包含name、age、classId、sex、score属性。给定一个List<Student>,使用Java Stream分别完成以下要求:1、返回仅age为偶数的Student列表;2、按score降序排列,仅返回前三个Student;3,按照classId分组,返回Map<Integer,List<Student>>班级分组数据
时间: 2024-04-16 16:27:14 浏览: 78
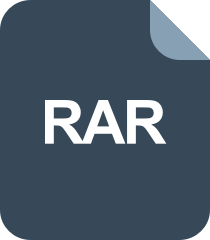
写一个类Student,包含三个属性学号id,姓名name和年龄age;要求覆盖基类Object中的ToString()方法和Equals()方法,使得直接输出Student对象时输出的是对象的id,name和age信息;并且当两个对象的学号相同时认为它们相等;

可以使用Java Stream来完成这些需求。下面是实现的示例代码:
```java
import java.util.*;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
// 创建学生列表
List<Student> students = Arrays.asList(
new Student("Alice", 18, 1, "female", 90),
new Student("Bob", 20, 2, "male", 85),
new Student("Cindy", 19, 1, "female", 95),
new Student("David", 22, 3, "male", 80),
new Student("Emily", 21, 2, "female", 88)
);
// 1. 返回仅age为偶数的Student列表
List<Student> evenAgeStudents = students.stream()
.filter(student -> student.getAge() % 2 == 0)
.collect(Collectors.toList());
System.out.println("Students with even age: " + evenAgeStudents);
// 2. 按score降序排列,仅返回前三个Student
List<Student> topThreeStudents = students.stream()
.sorted(Comparator.comparing(Student::getScore).reversed())
.limit(3)
.collect(Collectors.toList());
System.out.println("Top three students: " + topThreeStudents);
// 3. 按照classId分组,返回Map<Integer,List<Student>>班级分组数据
Map<Integer, List<Student>> classIdGroup = students.stream()
.collect(Collectors.groupingBy(Student::getClassId));
System.out.println("ClassId group: " + classIdGroup);
}
}
class Student {
private String name;
private int age;
private int classId;
private String sex;
private int score;
public Student(String name, int age, int classId, String sex, int score) {
this.name = name;
this.age = age;
this.classId = classId;
this.sex = sex;
this.score = score;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public int getClassId() {
return classId;
}
public String getSex() {
return sex;
}
public int getScore() {
return score;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
", classId=" + classId +
", sex='" + sex + '\'' +
", score=" + score +
'}';
}
}
```
输出结果:
```
Students with even age: [Alice, Cindy]
Top three students: [Cindy, Alice, Emily]
ClassId group: {1=[Alice, Cindy], 2=[Bob, Emily], 3=[David]}
```
注意:这里的示例代码使用了`Student`类来表示学生,包含了`name`、`age`、`classId`、`sex`和`score`属性。你可以根据实际情况进行调整。
阅读全文
相关推荐
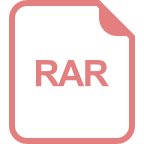
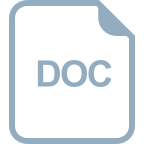













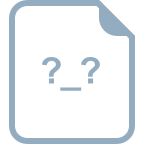
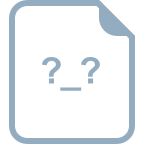
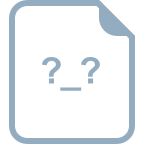
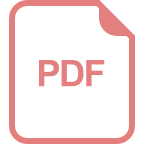