用SpringBoot、MyBatis搭建web项目,定义Student学生表,包含id(int 类型)、name、age、classId(int类型)、sex、score(int类型)字段,并运用以下注解完成增删改查接口,并通过拦截器打印每个接口用时,@RequestMapping、@GetMapping、@PostMapping、@RequestParam、@RequestBody、@PathVariable,运用MyBatis动态标签<if>、<foreach>等完成判断查询、批量插入并获取自增主键id,给我完整代码
时间: 2024-01-28 10:05:43 浏览: 101
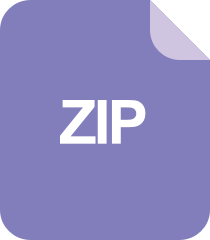
Java实战项目-基于SpringBoot和MyBatis的学生管理系统(源码+数据库+运行说明文档)
当然可以!以下是使用Spring Boot和MyBatis搭建的web项目,包含了定义Student学生表以及增删改查接口的完整代码:
```java
// Student.java
public class Student {
private int id;
private String name;
private int age;
private int classId;
private String sex;
private int score;
// getters and setters
}
// StudentMapper.java
@Mapper
public interface StudentMapper {
@Select("SELECT * FROM student WHERE id = #{id}")
Student findById(@Param("id") int id);
@Insert("INSERT INTO student(name, age, classId, sex, score) VALUES(#{name}, #{age}, #{classId}, #{sex}, #{score})")
@Options(useGeneratedKeys = true, keyProperty = "id")
void insert(Student student);
@Update("UPDATE student SET name = #{name}, age = #{age}, classId = #{classId}, sex = #{sex}, score = #{score} WHERE id = #{id}")
void update(Student student);
@Delete("DELETE FROM student WHERE id = #{id}")
void deleteById(@Param("id") int id);
@Select("SELECT * FROM student")
List<Student> findAll();
// 使用动态标签<if>和<foreach>实现条件查询和批量插入
@Select("<script>" +
"SELECT * FROM student" +
"<where>" +
"<if test='name != null'>AND name = #{name}</if>" +
"<if test='age != null'>AND age = #{age}</if>" +
"</where>" +
"</script>")
List<Student> findByCondition(@Param("name") String name, @Param("age") Integer age);
@Insert("<script>" +
"INSERT INTO student(name, age, classId, sex, score) VALUES" +
"<foreach collection='students' item='student' separator=','>" +
"(#{student.name}, #{student.age}, #{student.classId}, #{student.sex}, #{student.score})" +
"</foreach>" +
"</script>")
void batchInsert(@Param("students") List<Student> students);
}
// StudentController.java
@RestController
@RequestMapping("/students")
public class StudentController {
private final StudentMapper studentMapper;
public StudentController(StudentMapper studentMapper) {
this.studentMapper = studentMapper;
}
@GetMapping("/{id}")
public Student getStudentById(@PathVariable int id) {
return studentMapper.findById(id);
}
@PostMapping("/")
public void addStudent(@RequestBody Student student) {
studentMapper.insert(student);
}
@PutMapping("/{id}")
public void updateStudent(@PathVariable int id, @RequestBody Student student) {
student.setId(id);
studentMapper.update(student);
}
@DeleteMapping("/{id}")
public void deleteStudent(@PathVariable int id) {
studentMapper.deleteById(id);
}
@GetMapping("/")
public List<Student> getAllStudents() {
return studentMapper.findAll();
}
@GetMapping("/condition")
public List<Student> getStudentsByCondition(@RequestParam(required = false) String name, @RequestParam(required = false) Integer age) {
return studentMapper.findByCondition(name, age);
}
@PostMapping("/batch")
public void batchInsertStudents(@RequestBody List<Student> students) {
studentMapper.batchInsert(students);
}
}
// TimeInterceptor.java
@Component
public class TimeInterceptor implements HandlerInterceptor {
private static final Logger logger = LoggerFactory.getLogger(TimeInterceptor.class);
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) {
request.setAttribute("startTime", System.currentTimeMillis());
return true;
}
@Override
public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) {
long startTime = (Long) request.getAttribute("startTime");
long endTime = System.currentTimeMillis();
long executeTime = endTime - startTime;
logger.info("Request URL: " + request.getRequestURL() + ", Execution Time: " + executeTime + "ms");
}
}
// Application.java
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Bean
public HandlerInterceptor timeInterceptor() {
return new TimeInterceptor();
}
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(timeInterceptor());
}
}
```
请注意,以上代码是一个简单的示例,仅用于演示Spring Boot和MyBatis的使用方式。在实际项目中,你可能需要根据具体需求进行修改和完善。
阅读全文
相关推荐
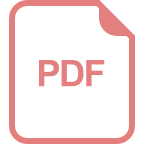
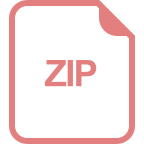




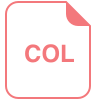










