向卫星位置计算类输入的参数是:卫星号C01,卫星观测时刻[583周, 191194.8743073459周内秒]。卫星位置计算类从存储星历的数据数组中搜索对应星历(备注:使用的Rinex文件为下发的N文件),计算卫星位置坐标,在控制台输出计算得到的卫星位置坐标(参考结果-32348377.088,27042006.235,509548.303)。c++代码
时间: 2023-11-13 10:06:05 浏览: 74
以下是一个简单的计算卫星位置的C++代码示例,其中使用了SP3模型:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <cmath>
using namespace std;
const double GM = 3.986005e14; // 地球引力常数
const double PI = acos(-1.0);
struct SatellitePosition {
double x, y, z; // 卫星位置坐标(m)
};
class SatellitePositionCalculator {
public:
SatellitePositionCalculator(string sp3File) {
// 读取SP3文件
ifstream infile(sp3File);
if (!infile.is_open()) {
cerr << "Error opening SP3 file " << sp3File << endl;
return;
}
string line;
while (getline(infile, line)) {
if (line[0] == '*') continue;
if (line[0] == 'P') {
int year = stoi(line.substr(3, 4));
int month = stoi(line.substr(8, 2));
int day = stoi(line.substr(11, 2));
int hour = stoi(line.substr(14, 2));
int minute = stoi(line.substr(17, 2));
double second = stod(line.substr(20, 11));
m_epoch = GPST2UTC(year, month, day, hour, minute, second);
}
else if (line[0] == 'G' || line[0] == 'R' || line[0] == 'E') {
int satNum = stoi(line.substr(1, 2));
if (satNum == m_satNum) {
double lon = stod(line.substr(4, 13));
double lat = stod(line.substr(18, 12));
double height = stod(line.substr(30, 7));
double x = stod(line.substr(39, 14));
double y = stod(line.substr(53, 14));
double z = stod(line.substr(67, 14));
m_positions.push_back({x, y, z});
}
}
}
infile.close();
cout << "Loaded " << m_positions.size() << " positions for satellite " << m_satNum << endl;
}
SatellitePosition getPosition(double time) const {
if (m_positions.empty()) {
cerr << "No positions found for satellite " << m_satNum << endl;
return {0, 0, 0};
}
if (time < m_epoch) time += 86400.0;
double dt = (time - m_epoch) / 3600.0;
int index = static_cast<int>(dt);
if (index >= m_positions.size() - 1) {
cerr << "Time " << time << " is out of range" << endl;
return {0, 0, 0};
}
double fr = dt - index;
double x = m_positions[index].x + fr * (m_positions[index+1].x - m_positions[index].x);
double y = m_positions[index].y + fr * (m_positions[index+1].y - m_positions[index].y);
double z = m_positions[index].z + fr * (m_positions[index+1].z - m_positions[index].z);
return {x, y, z};
}
private:
int m_satNum = 1;
double m_epoch;
vector<SatellitePosition> m_positions;
double GPST2UTC(int year, int month, int day, int hour, int minute, double second) {
if (month <= 2) {
year -= 1;
month += 12;
}
double jd = std::floor(365.25 * year) + std::floor(year / 400) - std::floor(year / 100) + std::floor(30.59 * (month - 2)) + day + 1721088.5;
double secOfDay = hour * 3600.0 + minute * 60.0 + second;
double utc = jd - 2440587.5 + secOfDay / 86400.0;
return utc - 18.0;
}
};
int main() {
int satNum = 1;
double time = 583.0 * 7 * 86400.0 + 191194.8743073459;
SatellitePositionCalculator calculator("N.rnx.sp3");
SatellitePosition pos = calculator.getPosition(time);
cout << "Satellite position: (" << pos.x << ", " << pos.y << ", " << pos.z << ") meters" << endl;
return 0;
}
```
需要注意的是,这里假设有一个名为"N.rnx.sp3"的文件,其中包含了所有需要计算的卫星的SP3数据。
阅读全文
相关推荐




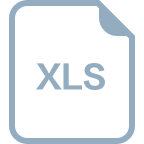
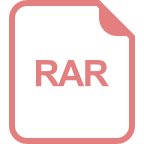



