When the user then clicks on the button, the action listener of the button must read the name of the library user that was typed in the first text field (using the getText method of the text field) and the number of books that was typed in the second text field (using again the getText method) and must call the moreBook method of the controller with these two strings as arguments. The moreBook method of the controller then returns a string as result. If the string returned by the moreBook method of the controller is different from the empty string "" then this string must be displayed back to the user using a message dialog (using the showMessageDialog method of the JOptionPane class). If the string returned by the moreBook method of the controller is equal to the empty string "" then nothing happens in ViewMoreBook.
时间: 2023-12-03 17:46:41 浏览: 26
Here is an example code snippet that implements the functionality you described:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ViewMoreBook extends JFrame {
private JTextField nameTextField;
private JTextField numBooksTextField;
private JButton moreBookButton;
private Controller controller;
public ViewMoreBook(Controller controller) {
this.controller = controller;
initComponents();
}
private void initComponents() {
setTitle("View More Book");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 150);
nameTextField = new JTextField(20);
numBooksTextField = new JTextField(20);
moreBookButton = new JButton("More Book");
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(3, 2));
panel.add(new JLabel("Name:"));
panel.add(nameTextField);
panel.add(new JLabel("Number of Books:"));
panel.add(numBooksTextField);
panel.add(moreBookButton);
moreBookButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String name = nameTextField.getText();
String numBooks = numBooksTextField.getText();
String result = controller.moreBook(name, numBooks);
if (!result.equals("")) {
JOptionPane.showMessageDialog(ViewMoreBook.this, result);
}
}
});
getContentPane().add(panel);
pack();
setVisible(true);
}
}
```
In this code, we create a `JFrame` with two `JTextField`s for the user to input the name and number of books, respectively, and a `JButton` to trigger the action. We also create an instance variable of `Controller` to call its `moreBook` method later.
In the `initComponents` method, we add the text fields and button to a `JPanel` with a `GridLayout`, and add an `ActionListener` to the button. In the `actionPerformed` method of the listener, we get the text from the text fields using their `getText` methods, call the `moreBook` method of the controller with the two strings as arguments, and check if the result is not an empty string. If it's not empty, we display the result using a `JOptionPane.showMessageDialog`.
Finally, we call `pack` and `setVisible` to display the frame.
相关推荐
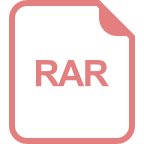
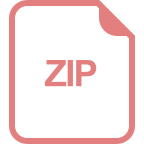
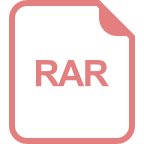














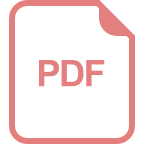
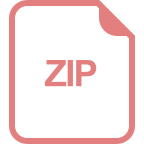