When the user then clicks on the button, the action listener of the button must read the name of the library user that was typed in the text field (using the getText method of the text field) and must call the getBook method of the controller with that user name as argument. The getBook method of the controller returns a string as result which must then be displayed back to the user using a message dialog (using the showMessageDialog method of the JOptionPane class). For example:
时间: 2023-11-28 20:51:25 浏览: 99
Sure, here's an example code that implements the functionality you described:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class LibraryGUI extends JFrame {
private JTextField userField;
private JButton getBookButton;
private Controller controller;
public LibraryGUI() {
super("Library GUI");
controller = new Controller(); // assuming you have a Controller class
// create components
userField = new JTextField(20);
getBookButton = new JButton("Get Book");
// add action listener to button
getBookButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String user = userField.getText();
String book = controller.getBook(user);
JOptionPane.showMessageDialog(null, book);
}
});
// add components to content pane
JPanel contentPane = (JPanel) getContentPane();
contentPane.setLayout(new FlowLayout());
contentPane.add(new JLabel("Enter library user:"));
contentPane.add(userField);
contentPane.add(getBookButton);
// set window properties
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
setLocationRelativeTo(null);
setVisible(true);
}
public static void main(String[] args) {
new LibraryGUI();
}
}
```
In this example, when the user clicks on the "Get Book" button, the action listener reads the name of the library user typed in the text field, calls the `getBook` method of the controller with that user name as argument, and displays the resulting string in a message dialog. Note that the `JOptionPane.showMessageDialog` method is used to display the message dialog.
阅读全文
相关推荐

















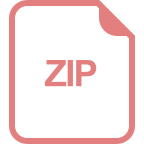
