如何实现以下需求:Dynamically generate random points by clicking in the graphical window and categorize these points into two classes.
时间: 2024-10-14 10:08:46 浏览: 32
To dynamically generate random points on a graphical window in MATLAB and categorize them into two classes, you can follow these steps:
1. **Set up the GUI**: First, create a new GUI with a `figure` or `uifigure` object. Add a `uicontrol` (like a `pushbutton`) to allow users to click, and another `axes` component for displaying the points.
```matlab
fig = uifigure('Name', 'Random Points Categorization');
ax = axes('Parent', fig);
btn = uibutton(fig, 'pushbutton', ...
'Position', [50 50 80 30], ...
'String', 'Add Point', ...
'ButtonPushedFcn', @(btn,event) addPoint(ax));
```
2. **AddPoint function**: Define a callback function that gets triggered when the button is clicked. Inside this function, generate a random point within the axes' limits and plot it with different symbols depending on which class it belongs to. You'll need to define a variable to keep track of the class assignments, e.g., an array of 0s and 1s.
```matlab
classAssignments = zeros(100, 1); % Assume initial capacity for 100 points
function addPoint(ax)
if isvalid(ax)
[x, y] = rand(1, 2); % Generate random coordinates
x = min(max(x, ax.XLim(1)), ax.XLim(2)); % Ensure within axes bounds
y = min(max(y, ax.YLim(1)), ax.YLim(2));
% Choose a random class (e.g., even numbers for one class, odd for the other)
class = mod(randi(2), 2);
hold on;
scatter(ax, x, y, [], classAssignments(end)); % Plot the point with appropriate symbol
classAssignments(end + 1) = class; % Update class assignment
legend(ax, {'Class 1', 'Class 2'}, 'Location', 'Best');
drawnow;
end
end
```
3. **Handle multiple clicks**: The current code only handles a single click. To allow multiple clicks, you might want to clear the plot after adding a new point and before allowing the next click:
```matlab
clearDataAndPlot = @(ax) scatter(ax, [], [], [], classAssignments);
btn.CallbackPostSet = @clearDataAndPlot;
```
Now, when you run your GUI and click the "Add Point" button, a new random point will be added, plotted with a unique symbol, and categorized based on the chosen random number.
**相关问题--:**
1. 如何处理GUI中的多个事件?
2. 如何在MATLAB中动态地改变图形上的点的颜色或符号?
3. 如何根据用户交互实时更新图表的图例?
阅读全文
相关推荐
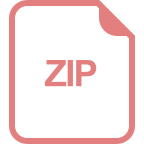
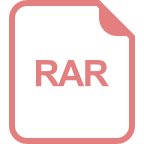
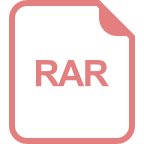














