您还可以创建代码来模拟Monty Hall问题。 创建一个名为random_door的函数,它使用numpy.random.choice从整数门索引列表(在本例中为1-3)中随机抽取1个门。使用此功能随机选择汽车后面的门和参赛者最初选择的门。 创建一个函数monty_choice,根据参赛者选择的门和带车的门来选择Monty打开的门。对于参赛者选择了带车的门的情况,通过使用n = 1的np.random.二项式函数模拟投掷均匀硬币来选择要打开的门。 创建一个函数win_car,根据所选择的策略、{switch, noswitch}、选手选择的门、带车的门和Monty打开的门来确定选手是否赢得汽车。 创建一个名为simulation的函数,允许您使用switch或noswitch策略运行n = 1000次模拟。 对每种可能的策略执行模拟。对于这两种策略,绘制并列条形图,显示每种策略的成功和失败数量。
时间: 2024-03-27 14:39:40 浏览: 21
以下是实现上述要求的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
def random_door():
return np.random.choice([1, 2, 3])
def monty_choice(car, contestant):
doors = [1, 2, 3]
doors.remove(car)
if contestant in doors:
doors.remove(contestant)
if contestant == car:
return np.random.binomial(1, 0.5, 1)[0] + 1
else:
return doors[0]
def win_car(car, contestant, monty, strategy):
if strategy == 'noswitch':
return contestant == car
elif strategy == 'switch':
doors = [1, 2, 3]
doors.remove(monty)
doors.remove(contestant)
contestant = doors[0]
return contestant == car
else:
print('Invalid strategy!')
return None
def simulation(strategy, n=1000):
car = random_door()
wins = 0
losses = 0
for i in range(n):
contestant = random_door()
monty = monty_choice(car, contestant)
if win_car(car, contestant, monty, strategy):
wins += 1
else:
losses += 1
return wins, losses
# run simulations for both switch and noswitch strategies
switch_wins, switch_losses = simulation('switch')
noswitch_wins, noswitch_losses = simulation('noswitch')
# plot the results
fig, ax = plt.subplots()
bar_width = 0.35
opacity = 0.8
index = np.arange(2)
rects1 = ax.bar(index, [switch_wins, noswitch_wins], bar_width,
alpha=opacity, color='b', label='Wins')
rects2 = ax.bar(index + bar_width, [switch_losses, noswitch_losses],
bar_width, alpha=opacity, color='r', label='Losses')
ax.set_xlabel('Strategy')
ax.set_ylabel('Count')
ax.set_title('Monty Hall Simulation Results')
ax.set_xticks(index + bar_width / 2)
ax.set_xticklabels(('Switch', 'No Switch'))
ax.legend()
plt.show()
```
这段代码首先定义了 `random_door()` 函数,使用 `numpy.random.choice()` 函数从整数门索引列表 `[1, 2, 3]` 中随机选择一个门。
接下来,我们定义了 `monty_choice()` 函数,该函数接受 `car` 和 `contestant` 作为输入,根据 Monty Hall 的规则选择 Monty 打开的门。如果选手选择了带车的门,那么 Monty 会随机选择两个门中的一个,这里我们使用 `numpy.random.binomial()` 函数模拟了这个随机过程。
然后,我们定义了 `win_car()` 函数,根据选手选择的门、带车的门和 Monty 打开的门来确定选手是否赢得了汽车。
最后,我们定义了 `simulation()` 函数,允许您运行 `n` 次模拟。对于每次模拟,我们选择一个带车的门,然后使用 `random_door()` 函数随机选择参赛者选择的门。然后,我们使用 `monty_choice()` 函数选择 Monty 打开的门。最后,我们使用 `win_car()` 函数确定参赛者是否赢得了汽车。
我们使用 `simulation()` 函数分别运行了 `switch` 和 `noswitch` 两种策略各 1000 次,并统计了成功和失败的次数。最后,我们绘制了一个并列条形图,显示了每种策略的成功和失败数量。
相关推荐
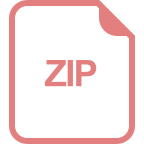
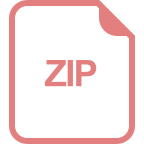















