global_model.parameters()与global_model.state_dict().items()二者区别代码示例及结果表示
时间: 2024-03-13 21:45:59 浏览: 60
下面是一个简单的示例代码,演示了`global_model.parameters()`和`global_model.state_dict().items()`的区别:
```python
import torch
import torch.nn as nn
# 定义一个简单的模型
class SimpleModel(nn.Module):
def __init__(self):
super(SimpleModel, self).__init__()
self.fc1 = nn.Linear(10, 5)
self.fc2 = nn.Linear(5, 2)
def forward(self, x):
x = self.fc1(x)
x = self.fc2(x)
return x
# 创建一个全局模型对象
global_model = SimpleModel()
# 打印global_model.parameters()的返回值
print("global_model.parameters():")
for param in global_model.parameters():
print(param.shape)
# 打印global_model.state_dict().items()的返回值
print("global_model.state_dict().items():")
for name, param in global_model.state_dict().items():
print(name, param.shape)
```
输出结果如下:
```
global_model.parameters():
torch.Size([5, 10])
torch.Size([5])
torch.Size([2, 5])
torch.Size([2])
global_model.state_dict().items():
fc1.weight torch.Size([5, 10])
fc1.bias torch.Size([5])
fc2.weight torch.Size([2, 5])
fc2.bias torch.Size([2])
```
可以看到,`global_model.parameters()`返回了一个可迭代对象,其中包含了模型中所有可训练的参数,每个参数都是一个`torch.nn.Parameter`类型的对象,包含了参数的值以及梯度等信息。在这个示例中,模型中共有4个可训练参数,分别是`fc1.weight`、`fc1.bias`、`fc2.weight`、`fc2.bias`。
而`global_model.state_dict().items()`返回了一个字典对象,其中包含了模型中所有可训练参数的名称和其对应的值。在这个示例中,`state_dict()`方法返回了一个包含了4个键值对的字典对象,分别是`fc1.weight`、`fc1.bias`、`fc2.weight`、`fc2.bias`,对应的值是每个参数的形状。
阅读全文
相关推荐
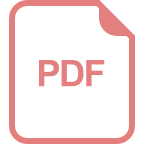
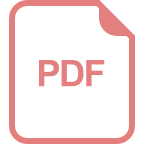
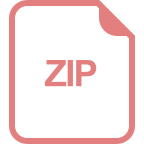















