用C语言请你帮助班级编写一个学生成绩输出函数print,能够输出学生的信息和成绩。每个记录包括:学号num、姓名name、成绩数组score[3],用主函数输入这些记录,用print函数输出这些记录。为了不失一般性,用#define定义学生数量,验证中以5名学生为例。 函数原型:void print ( struct student stu[] ) 程序运行结果示例: Input the score information of student 1: No.: 101↙ Name: LiBo↙ Sco
时间: 2024-03-03 12:49:57 浏览: 91
下面是实现你所需功能的代码:
```c
#include <stdio.h>
#define NUM_OF_STUDENTS 5
struct student {
int num;
char name[20];
int score[3];
};
void print(struct student stu[]);
int main() {
struct student stu[NUM_OF_STUDENTS];
for (int i = 0; i < NUM_OF_STUDENTS; i++) {
printf("Input the score information of student %d:\n", i + 1);
printf("No.: ");
scanf("%d", &stu[i].num);
printf("Name: ");
scanf("%s", stu[i].name);
for (int j = 0; j < 3; j++) {
printf("Score %d: ", j + 1);
scanf("%d", &stu[i].score[j]);
}
}
print(stu);
return 0;
}
void print(struct student stu[]) {
printf("No.\tName\tScore1\tScore2\tScore3\n");
for (int i = 0; i < NUM_OF_STUDENTS; i++) {
printf("%d\t%s\t%d\t%d\t%d\n", stu[i].num, stu[i].name, stu[i].score[0], stu[i].score[1], stu[i].score[2]);
}
}
```
解释一下代码各部分的作用:
1. 定义一个结构体 `student`,包含三个成员变量:学号 `num`、姓名 `name` 和成绩数组 `score`。
2. 通过 `#define` 定义学生数量 `NUM_OF_STUDENTS`,这里定义为 5。
3. 定义函数 `print` 的原型: `void print(struct student stu[])`,接收一个结构体数组 `stu`。
4. 在 `main` 函数中,定义一个长度为 `NUM_OF_STUDENTS` 的结构体数组 `stu`,通过循环依次读入每个学生的信息。
5. 调用函数 `print` 输出每个学生的信息。
6. 实现函数 `print`,利用循环遍历结构体数组 `stu`,依次输出每个学生的信息和成绩。
阅读全文
相关推荐
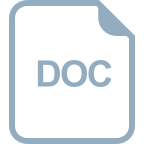
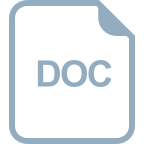
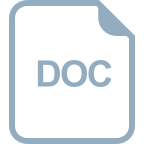
















