改为C++语言并且手动输入权重图
时间: 2024-12-14 19:27:21 浏览: 3
在C++中实现Dijkstra算法处理手动输入权重图,我们可以先创建一个邻接表结构来存储图,并通过用户输入来填充权重。下面是一个简单的C++版本:
```cpp
#include <iostream>
#include <vector>
#include <queue>
#include <climits>
// 定义图节点
struct Node {
int id;
std::unordered_map<int, int> neighbors; // 存储相邻节点及其权重
};
// 使用优先队列实现Dijkstra算法
void dijkstra(const std::vector<Node>& nodes, int start) {
std::priority_queue<std::pair<int, int>, std::vector<std::pair<int, int>>, std::greater<std::pair<int, int>>> pq;
std::vector<int> distances(nodes.size(), INT_MAX); // 初始化所有节点的距离为无穷大
distances[start] = 0;
pq.push({0, start});
while (!pq.empty()) {
int currentDistance, currentNodeId;
tie(currentDistance, currentNodeId) = pq.top();
pq.pop();
if (currentDistance > distances[currentNodeId])
continue; // 已经更新过的更优路径不会再次加入
for (const auto& [neighborId, weight] : nodes[currentNodeId].neighbors) {
int newDistance = currentDistance + weight;
if (newDistance < distances[neighborId]) {
distances[neighborId] = newDistance;
pq.push({newDistance, neighborId});
}
}
}
for (int i = 0; i < nodes.size(); ++i) {
if (distances[i] == INT_MAX)
std::cout << "No path found to node " << i << std::endl;
else
std::cout << "Shortest distance from " << start << " to " << i << ": " << distances[i] << std::endl;
}
}
int main() {
std::vector<Node> nodes;
int numNodes, numEdges, startNode;
std::cin >> numNodes >> numEdges >> startNode; // 输入节点数、边数和起始节点
for (int i = 0; i < numNodes; ++i) {
nodes.push_back(Node{i});
}
for (int i = 0; i < numEdges; ++i) {
int u, v, w;
std::cin >> u >> v >> w; // 输入两个节点和它们之间的权重
nodes[u - 1].neighbors[v - 1] = w;
nodes[v - 1].neighbors[u - 1] = w; // 对称边
}
dijkstra(nodes, startNode - 1);
return 0;
}
```
这个程序首先创建节点和边,然后通过循环读取用户输入来构建邻接表。注意,C++中的索引是从0开始的,所以需要减去1来对应题目描述中的1-indexed节点。
阅读全文
相关推荐



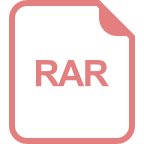



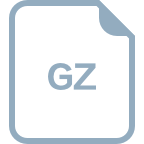










