#include <bits/stdc++.h> using namespace std; int main() { char s[]="hello C++ world"; char *d; char c[]=" "; d=&c; char *p; p=strtok(s,d); while (p!=NULL) { cout <<p <<endl; p=strtok(NULL,d); } return 0; }
时间: 2024-03-10 12:48:28 浏览: 91
在这段代码中,你尝试将指向字符数组的指针 `&c` 赋值给字符指针 `d`,这是不允许的,因为两者类型不同。正确的做法是将 `d` 声明为字符数组类型,即 `char d[] = " "`。这样就可以将空格字符数组的地址赋值给 `d`。修改后的代码如下:
```
#include <bits/stdc++.h>
using namespace std;
int main() {
char s[] = "hello C++ world";
char d[] = " ";
char *p;
p = strtok(s, d);
while (p != NULL) {
cout << p << endl;
p = strtok(NULL, d);
}
return 0;
}
```
这段代码的功能是将字符串 `s` 按照空格进行分割,并输出分割后的结果。注意,这段代码使用了 `strtok()` 函数进行字符串分割操作。
相关问题
#include<bits/stdc++.h> using namespace std; int main(){ char s; while(cin>>s,s!='\0'){ cout<<s; } return 0; }为什么循环没有停止?
这段 C++ 代码是一个简单的程序,它通过无限循环读取用户输入并逐个打印字符,直到遇到空字符('\0')为止。`while` 循环条件 `cin >> s, s != '\0'` 的逻辑是这样的:
1. 当从标准输入 (`cin`) 中读入一个字符赋值给 `s` 后,`cin >> s` 会继续等待用户输入下一个字符,除非按下 Enter 键或遇到非字符输入。
2. 如果输入的是一个非空字符(包括空格、数字或其他特殊字符),`s` 将不会等于空字符,所以循环将继续。
3. 只有当用户输入了一个空字符('\0'通常出现在字符串结束时),条件才会变为 `false`,循环才会终止。
因此,如果用户始终未输入空字符就关闭了程序或程序没有提供其他退出机制,这个循环就会一直运行下去,直到手动停止。为了避免这种无限制的循环,可以在循环内部添加一个条件检查或在适当的时候显式地设置一个退出标志,比如添加一个计数器或判断一个特定的结束标志。例如:
```cpp
#include <bits/stdc++.h>
using namespace std;
int main() {
char s;
bool stop = false; // 添加一个标志用于控制循环
while (cin >> s, !stop) { // 修改循环条件
if (s == '\0') {
stop = true; // 输入空字符时,设置停止标志
} else {
cout << s;
}
}
return 0;
}
``` #include<bits/stdc++.h> using namespace std; int main() { char s[10000000]; getline(s,cin); } ```
该C++代码中存在两个主要问题:
1. 未包含`#include <string>`:`getline()`函数需要`<string>`库来正确工作。
2. 传递给`getline()`的参数类型不匹配:`getline()`通常用于读取字符串,但在这里你试图将一个字符数组`s`传递给它,而不是一个引用或指针到`std::string`对象。
修复这两个问题后,完整的代码如下:
```cpp
#include <bits/stdc++.h>
#include <string> // 添加对<string>库的包含
using namespace std;
int main()
{
string s; // 使用std::string而非char数组
getline(cin, s); // 使用cin并传入string类型的变量s
return 0;
}
```
错误分析:
- 错误1:`#include <string>` 缺失,导致无法使用`std::string`类及其相关函数(如`getline()`)。
- 错误2:将`char s[10000000];`改为`string s;`以适应`getline()`,因为getline()是用于读取字符串的,而字符数组不能直接作为其参数。
修复后的代码解决了这些问题,并且可以正常从标准输入读取一行文本。
阅读全文
相关推荐
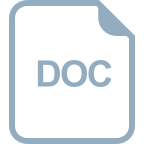
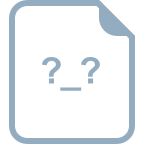













