// 创建结果标签 resultLabel = new JLabel(); resultLabel.setBounds(160, 100, 300, 100); //resultLabel.setHorizontalAlignment(SwingConstants.CENTER); resultLabel.setFont(new Font("宋体", Font.PLAIN, 16)); gamePanel.add(resultLabel);怎么扩大标签的行数,使它容纳更多的字
时间: 2024-03-22 08:37:51 浏览: 50
要扩大标签的行数,可以使用JLabel的setPreferredSize()方法或者setMaximumSize()方法设置标签的大小。同时,需要将JLabel的换行属性设置为true,使用setLineWrap()和setWrapStyleWord()方法实现。例如:
```
resultLabel = new JLabel();
resultLabel.setBounds(160, 100, 300, 100);
resultLabel.setLineWrap(true);
resultLabel.setWrapStyleWord(true);
resultLabel.setPreferredSize(new Dimension(300, 200)); //设置标签大小
resultLabel.setFont(new Font("宋体", Font.PLAIN, 16));
gamePanel.add(resultLabel);
```
这样就可以将标签的行数扩大,使其容纳更多的字。
相关问题
java这段代码private void CreateMainWindow(){ this.setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE); WindowBar = new JMenuBar(); this.setJMenuBar(WindowBar); this.setTitle("停车场管理系统"); int WindowHeight = 300; int WindowWidth = 400; Dimension Screen = Toolkit.getDefaultToolkit().getScreenSize(); int ScreenWidth = Screen.width; int ScreenHeight = Screen.height; this.setResizable(false); this.setLayout(null); this.setBounds((ScreenWidth-WindowWidth)/2,(ScreenHeight-WindowHeight)/2,WindowWidth,WindowHeight); jLabelTitle = new JLabel(); getContentPane().add(jLabelTitle); jLabelTitle.setText("欢迎进入"); jLabelTitle.setBounds(130, 50, 141, 30); //jLabelTitle.setLocation(100, 20); jLabelTitle.setFont(new java.awt.Font("宋体",1,20)); jLabelTitle.setHorizontalAlignment(SwingConstants.CENTER); jLabel = new JLabel(); getContentPane().add(jLabel); getContentPane().add(jLabelTitle); jLabel.setText("停车场信息管理系统"); jLabel.setBounds(50, 100, 300, 30); jLabel.setFont(new java.awt.Font("宋体",1,30)); jLabel.setHorizontalAlignment(SwingConstants.CENTER); notMenu = new JMenu("管理模式"); UserMenu = new JMenu("信息管理"); TypeMenu = new JMenu("车位管理"); WindowBar.add(UserMenu); WindowBar.add(notMenu); WindowBar.add(TypeMenu); JMenuItem AddItem ,DeleteItem,ChangeItem,QueryItem; AddItem = new JMenuItem("添加"); DeleteItem = new JMenuItem("删除"); ChangeItem = new JMenuItem("修改"); QueryItem = new JMenuItem("查询"); notMenu.add(AddItem); notMenu.add(DeleteItem); notMenu.add(ChangeItem); notMenu.add(QueryItem); AddItem.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { // TODO Auto-generated method stub //MainWindow.this.setVisible(false); AddFrame add = new AddFrame(); add.setVisible(true); } }); QueryItem.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { // TODO Auto-generated method stub //MainWindow.this.setVisible(false); QueryFrame query = new QueryFrame(); query.setVisible(true); } }); }怎么设置点击按钮打开另一个窗口,该如何修改
这段代码定义了一个名为"CreateMainWindow"的方法,该方法创建了一个主窗口,并在主窗口中添加了菜单栏、标签、菜单项等元素。
如果你想点击按钮打开另一个窗口,可以在代码中添加一个按钮,然后按照以下步骤操作:
1. 定义一个按钮,并将其添加到主窗口的内容面板中。
2. 为按钮添加一个"ActionListener"事件监听器,在事件发生时,打开另一个窗口。
例如,你可以在代码中添加一个按钮,如下所示:
```
JButton button = new JButton("打开窗口");
getContentPane().add(button);
```
然后,为按钮添加"ActionListener"事件监听器,如下所示:
```
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// TODO Auto-generated method stub
// 打开另一个窗口
OtherWindow window = new OtherWindow();
window.setVisible(true);
}
});
```
在这里,"OtherWindow"是另一个窗口的类名,在点击按钮时,会创建一个"OtherWindow"对象,并调用其"setVisible"方法使其可见。
希望这能帮到你!
import tkinter as tk import random as ra import threading as td import time as ti def Love(): root=tk.Tk() width=200 height=50 screenwidth=root.winfo_screenwidth() screenheight=root.winfo_screenheight() x=ra.randint(0,screenwidth) y=ra.randint(0,screenheight) root.title("❤") root.geometry("%dx%d+%d+%d"%(width,height,x,y)) tk.Label(root,text='I LOVE YOU!',fg='white',bg='pink',font=("Comic Sans MS",15),width=30,height=5).pack() root.mainloop() def Heart(): root=tk.Tk() screenwidth=root.winfo_screenwidth() screenheight=root.winfo_screenheight() width=600 height=400 x=(screenwidth-width)//2 y=(screenheight-height)//2 root.title("❤") root.geometry("%dx%d+%d+%d"%(screenwidth,screenheight,0,0)) tk.Label(root,text='❤',fg='pink',bg='white',font=("Comic Sans MS",500),width=300,height=20).pack() root.mainloop() t=td.Thread(target=Heart) t.start() for i in range(50): t=td.Thread(target=Love) ti.sleep(0.1) t.start()用java的swing实现
我可以告诉你如何用Java Swing实现类似的效果,但是代码实现可能会与您在Python中使用的代码有所不同。以下是一个简单的Java Swing示例,可以在屏幕上显示一些爱心和一些文本:
```java
import java.awt.*;
import javax.swing.*;
public class LoveFrame extends JFrame {
public LoveFrame() {
// 设置窗口大小
setPreferredSize(new Dimension(600, 400));
// 将窗口放置在屏幕中央
setLocationRelativeTo(null);
// 设置窗口标题
setTitle("❤");
// 设置窗口关闭时退出程序
setDefaultCloseOperation(EXIT_ON_CLOSE);
// 添加一个文本标签
JLabel label = new JLabel("I LOVE YOU!");
label.setForeground(Color.WHITE);
label.setBackground(Color.PINK);
label.setFont(new Font("Comic Sans MS", Font.PLAIN, 15));
label.setHorizontalAlignment(SwingConstants.CENTER);
label.setVerticalAlignment(SwingConstants.CENTER);
label.setOpaque(true);
getContentPane().add(label, BorderLayout.CENTER);
// 添加一些爱心
getContentPane().setBackground(Color.WHITE);
setLayout(null);
for (int i = 0; i < 50; i++) {
int x = (int) (Math.random() * getWidth());
int y = (int) (Math.random() * getHeight());
LoveIcon icon = new LoveIcon();
JLabel heart = new JLabel(icon);
heart.setBounds(x, y, icon.getIconWidth(), icon.getIconHeight());
getContentPane().add(heart);
}
// 显示窗口
pack();
setVisible(true);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new LoveFrame();
}
});
}
// 爱心图标
private static class LoveIcon implements Icon {
private static final int SIZE = 20;
private static final int[] X_POINTS = { 20, 22, 28, 26, 20, 14, 12, 10, 2, 0, 6, 10 };
private static final int[] Y_POINTS = { 0, 4, 2, 8, 16, 8, 2, 4, 0, 10, 16, 10 };
private static final Polygon POLYGON = new Polygon(X_POINTS, Y_POINTS, X_POINTS.length);
public int getIconWidth() {
return SIZE;
}
public int getIconHeight() {
return SIZE;
}
public void paintIcon(Component c, Graphics g, int x, int y) {
g.setColor(Color.PINK);
g.fillPolygon(POLYGON);
}
}
}
```
这段代码使用Java Swing API绘制了一个窗口,其中包括一个文本标签和一些爱心。要使用此代码,请将其保存为名为“LoveFrame.java”的文件,然后使用Java编译器将其编译为可执行的Java类。
阅读全文
相关推荐
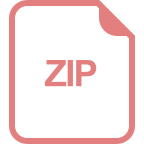
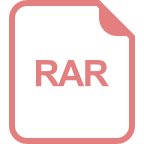
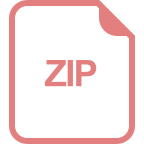
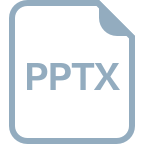
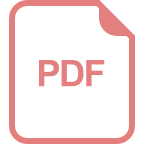
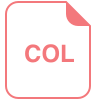





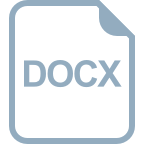
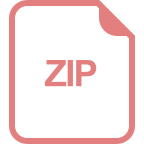
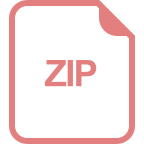
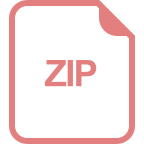