将字符串中的小写字母转换成大写字母c++
时间: 2023-08-28 11:38:04 浏览: 199
可以使用 C++ 中的标准库函数 `toupper`,它可以将小写字母转换成大写字母。具体实现如下:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello, World!";
for (int i = 0; i < str.size(); i++) {
if (islower(str[i])) {
str[i] = toupper(str[i]);
}
}
cout << str << endl; // 输出 HELLO, WORLD!
return 0;
}
```
在上面的代码中,我们先判断字符串中每个字符是否为小写字母,如果是,则使用 `toupper` 将其转换为大写字母。最后输出转换后的字符串。
相关问题
用C++编写程序,输入大小写字母混合的字符串,将大写字母转换为小写字母,将小写字母转换为大写字母。然后,输出转换以后的字符串。
在C++中,你可以使用标准库中的`<cctype>`头文件提供的`tolower()`和`toupper()`函数来实现字符的大小写转换。下面是一个简单的示例程序:
```cpp
#include <iostream>
#include <string>
#include <cctype> // 包含tolower()和toupper()
std::string convertCase(const std::string& input) {
std::string output;
for (char c : input) {
if (islower(c)) { // 如果是小写字母
output += toupper(c);
} else if (isupper(c)) { // 如果是大写字母
output += tolower(c);
} else { // 其他字符不变
output += c;
}
}
return output;
}
int main() {
std::string str;
std::cout << "请输入一个大小写字母混合的字符串: ";
std::getline(std::cin, str); // 使用getline获取带空格的字符串
std::string convertedStr = convertCase(str);
std::cout << "转换后的字符串: " << convertedStr << std::endl;
return 0;
}
```
在这个程序中,我们首先读取用户输入的字符串,然后遍历每个字符,如果它是小写字母,就转换为大写;如果是大写字母,就转换为小写;其他字符保持原样。最后,返回转换后的字符串并输出。
C++代码解决统计字符串中大小写字母数量的最小值。它使用两个列表分别记录字符串中小写字母和大写字母的数量,并遍历字符串中的每个字符来更新这两个列表。接着,它遍历字符串中的每个位置,计算在该位置之前的小写字母数量和在该位置之后的大写字母数量。最后,它返回所有位置上计算出的值的最小值,这个最小值就是字符串中大小写字母数量的最小值。
下面是C++代码实现:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
int min_count(string s) {
int n = s.length(); // 获取字符串长度
vector<int> lowercase(n + 1), uppercase(n + 1); // 初始化小写字母和大写字母数量列表
for (int i = 1; i <= n; i++) {
lowercase[i] = lowercase[i - 1] + islower(s[i - 1]); // 更新小写字母数量列表
uppercase[i] = uppercase[i - 1] + isupper(s[i - 1]); // 更新大写字母数量列表
}
int ans = INT_MAX; // 初始化最小值为INT_MAX
for (int i = 0; i <= n; i++) {
ans = min(ans, lowercase[i] + uppercase[n] - uppercase[i]); // 计算最小值
}
return ans; // 返回最小值
}
int main() {
string s = "aAbbCcD";
cout << min_count(s) << endl; // 输出 2
return 0;
}
```
这段代码首先定义了一个函数`int min_count(string s)`,它接收一个字符串`s`作为输入,并返回一个整数。函数内部使用`islower()`和`isupper()`方法来判断字符是否是小写字母和大写字母,进而更新两个列表`lowercase`和`uppercase`,这两个列表记录了字符串中每个位置之前的小写字母数量和每个位置之后的大写字母数量。接着,它遍历了字符串中的每个位置,并计算在该位置之前的小写字母数量和在该位置之后的大写字母数量。最后,它返回所有位置上计算出的值的最小值,这个最小值就是字符串中大小写字母数量的最小值。
在主函数中,我们可以测试一下这个函数。
阅读全文
相关推荐
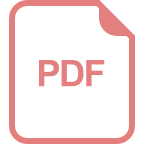
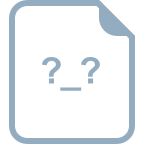












