C++查找轮廓端点,如果端点之间的距离小于阈值则进行连接
时间: 2023-07-08 13:04:44 浏览: 128
实现这个功能需要用到OpenCV库中的函数,具体步骤如下:
1. 对图像进行二值化处理,得到轮廓。
2. 对轮廓进行查找,可以使用`findContours`函数。
3. 遍历每一个轮廓,对于每一个轮廓,找到它的端点,并保存在`vector`中,可以使用`approxPolyDP`函数和`convexHull`函数。
4. 遍历每一个端点,计算它与其他端点之间的距离,如果小于阈值则进行连接,可以使用`line`函数。
下面给出代码示例:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
#include <vector>
using namespace cv;
using namespace std;
const int THRESHOLD = 30; // 阈值
int main()
{
Mat src = imread("test.png");
Mat gray, binary;
cvtColor(src, gray, COLOR_BGR2GRAY);
threshold(gray, binary, 0, 255, THRESH_BINARY | THRESH_OTSU);
vector<vector<Point>> contours;
findContours(binary, contours, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
for (int i = 0; i < contours.size(); i++) {
vector<Point> hull;
approxPolyDP(contours[i], hull, 10, true);
if (hull.size() < 3) continue;
vector<Point> endpoints;
for (int j = 0; j < hull.size(); j++) {
float dist = 0;
for (int k = 0; k < hull.size(); k++) {
if (j == k) continue;
float d = norm(hull[j] - hull[k]);
if (d < dist || dist == 0) dist = d;
}
if (dist > THRESHOLD) endpoints.push_back(hull[j]);
}
if (endpoints.size() < 2) continue;
for (int j = 0; j < endpoints.size(); j++) {
for (int k = j + 1; k < endpoints.size(); k++) {
line(src, endpoints[j], endpoints[k], Scalar(0, 0, 255), 2);
}
}
}
imshow("result", src);
waitKey(0);
return 0;
}
```
其中,`approxPolyDP`函数和`convexHull`函数用于查找轮廓的端点,`line`函数用于连接端点。需要注意的是,`approxPolyDP`函数和`convexHull`函数的参数需要根据实际情况进行调整。
阅读全文
相关推荐








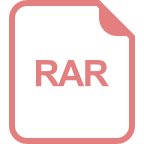







