vue3+ts 项目,scripts标签处已有setup,如何实现点击页面中的某一按钮在当前页面出现弹窗并以表格形式展示弹窗内容,且弹窗可点击确认和取消
时间: 2024-04-16 10:28:30 浏览: 25
在Vue3+TypeScript项目中,你可以使用Vue的弹窗组件以及表格组件来实现你的需求。
首先,在你的Vue组件中,你可以使用`<button>`元素来表示按钮,然后使用Vue的`v-on`指令来监听按钮的点击事件。当按钮被点击时,你可以在事件处理函数中触发弹窗的显示。
接下来,你需要在当前页面中创建一个弹窗组件,并在需要显示弹窗的时候将其渲染到页面上。你可以使用Vue的条件渲染指令`v-if`来控制弹窗的显示和隐藏。同时,你可以在弹窗组件中使用表格组件来展示内容。
最后,你可以在弹窗组件中添加确认和取消按钮,并通过绑定事件处理函数来实现点击确认和取消按钮时的操作。
下面是一个简单的实现示例:
```html
<template>
<div>
<button @click="showPopup">点击打开弹窗</button>
<div v-if="isPopupVisible" class="popup">
<table>
<!-- 表格内容 -->
</table>
<div class="buttons">
<button @click="confirm">确认</button>
<button @click="cancel">取消</button>
</div>
</div>
</div>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue';
export default defineComponent({
setup() {
const isPopupVisible = ref(false);
const showPopup = () => {
isPopupVisible.value = true;
};
const confirm = () => {
// 点击确认按钮后的操作
isPopupVisible.value = false;
};
const cancel = () => {
// 点击取消按钮后的操作
isPopupVisible.value = false;
};
return {
isPopupVisible,
showPopup,
confirm,
cancel,
};
},
});
</script>
<style>
.popup {
/* 弹窗样式 */
}
.buttons {
/* 按钮样式 */
}
</style>
```
在上面的示例中,当点击按钮时,`showPopup`函数会将`isPopupVisible`的值设置为`true`,从而显示弹窗。弹窗中的确认按钮和取消按钮分别绑定了`confirm`和`cancel`函数,点击这两个按钮时会执行对应的操作,并将`isPopupVisible`的值设置为`false`,从而隐藏弹窗。
你可以根据实际需求,修改弹窗组件和表格内容的样式,并根据业务逻辑来实现确认和取消按钮的具体操作。
相关推荐
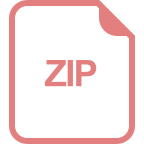
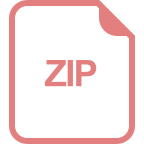














