图书管理系统c++代码
时间: 2024-01-07 17:22:53 浏览: 28
根据提供的引用内容,以下是一个简单的图书管理系统的C++代码示例:
```cpp
#include <iostream>
#include <vector>
#include <fstream>
using namespace std;
class CBook {
private:
string title;
string author;
int quantity;
public:
CBook(string t, string a, int q) {
title = t;
author = a;
quantity = q;
}
string getTitle() {
return title;
}
string getAuthor() {
return author;
}
int getQuantity() {
return quantity;
}
};
class CUser {
protected:
string name;
public:
CUser(string n) {
name = n;
}
string getName() {
return name;
}
};
class CStudent : public CUser {
public:
CStudent(string n) : CUser(n) {}
};
class CTeacher : public CUser {
public:
CTeacher(string n) : CUser(n) {}
};
class CAdministrator {
public:
void addBook(vector<CBook>& books, string title, string author, int quantity) {
CBook newBook(title, author, quantity);
books.push_back(newBook);
}
void viewBorrowers(vector<CUser>& users) {
cout << "Current borrowers:" << endl;
for (CUser user : users) {
cout << user.getName() << endl;
}
}
void viewBooks(vector<CBook>& books) {
cout << "Current books:" << endl;
for (CBook book : books) {
cout << "Title: " << book.getTitle() << ", Author: " << book.getAuthor() << ", Quantity: " << book.getQuantity() << endl;
}
}
};
class CManagementBooks {
public:
void borrowBook(vector<CBook>& books, vector<CUser>& users, string bookTitle, string userName) {
bool bookFound = false;
bool userFound = false;
for (CBook& book : books) {
if (book.getTitle() == bookTitle && book.getQuantity() > 0) {
bookFound = true;
book.quantity--;
break;
}
}
for (CUser& user : users) {
if (user.getName() == userName) {
userFound = true;
break;
}
}
if (bookFound && userFound) {
cout << "Book borrowed successfully!" << endl;
} else {
cout << "Book or user not found, or book is not available." << endl;
}
}
void returnBook(vector<CBook>& books, vector<CUser>& users, string bookTitle, string userName) {
bool bookFound = false;
bool userFound = false;
for (CBook& book : books) {
if (book.getTitle() == bookTitle) {
bookFound = true;
book.quantity++;
break;
}
}
for (CUser& user : users) {
if (user.getName() == userName) {
userFound = true;
break;
}
}
if (bookFound && userFound) {
cout << "Book returned successfully!" << endl;
} else {
cout << "Book or user not found." << endl;
}
}
};
int main() {
vector<CBook> books;
vector<CUser> users;
CAdministrator admin;
CManagementBooks bookManager;
// 添加书籍
admin.addBook(books, "Book 1", "Author 1", 5);
admin.addBook(books, "Book 2", "Author 2", 3);
admin.addBook(books, "Book 3", "Author 3", 2);
// 添加用户
CStudent student("John");
CTeacher teacher("Jane");
users.push_back(student);
users.push_back(teacher);
// 借书
bookManager.borrowBook(books, users, "Book 1", "John");
bookManager.borrowBook(books, users, "Book 2", "Jane");
// 还书
bookManager.returnBook(books, users, "Book 1", "John");
// 查看当前借阅情况和图书信息
admin.viewBorrowers(users);
admin.viewBooks(books);
return 0;
}
```
相关推荐
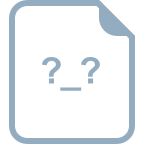
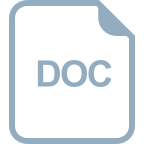













