n = int(input()) m = list(map(int, input().split())) cnt = 1 ans = 1 for i in range(len(m)): if m[i+1] == m[i] + 1: cnt+=1 if cnt > ans: ans = cnt else: cnt = 0 print(ans)优化代码,解决list out of index
时间: 2024-04-12 14:29:45 浏览: 124
你可以尝试在循环遍历列表时,将循环条件改为 `range(len(m)-1)`,这样可以避免出现索引溢出的情况。另外,你可以将 `ans` 变量的初始化值设置为 0,这样可以正确处理列表中不存在连续递增序列的情况。以下是优化后的代码:
```python
n = int(input())
m = list(map(int, input().split()))
cnt = 1
ans = 0
for i in range(len(m)-1):
if m[i+1] == m[i] + 1:
cnt += 1
if cnt > ans:
ans = cnt
else:
cnt = 1
print(ans)
```
这样,你就可以避免出现索引溢出的问题,并正确计算连续递增序列的最大长度。
相关问题
纠错import bisect def min_operations(a, b): f = [0] * (n + 1) for i in range(1, n + 1): j = i - 1 while j > 0 and a[j] > b[i - 1]: j -= 1 f[i] = f[i - 1] + (i - j - 1) ans = float('inf') for i in range(n): cnt = i + 1 + n - bisect.bisect_left(b, a[i] + 1) ans = min(ans, cnt + f[n] - f[i + 1]) return ans t = int(input()) for _ in range(t): n = int(input()) a = list(map(int, input().split())) b = list(map(int, input().split())) print(min_operations(a, b))
这段代码的主要问题是没有定义变量 `n`,因此在第 3 行代码中会抛出 `NameError: name 'n' is not defined` 异常。
为了解决这个问题,我们需要将变量 `n` 的定义提前到函数外部,在函数中使用 `n` 时,需要使用 `global` 关键字声明它是全局变量。
此外,在第 16 行代码中,应该将 `a[i] + 1` 改为 `b[i] + 1`。这是因为我们要找到 `b` 中第一个大于 `a[i]` 的元素,而不是 `a[i] + 1`。
修改后的代码如下:
```python
import bisect
n = 0
def min_operations(a, b):
global n
f = [0] * (n + 1)
for i in range(1, n + 1):
j = i - 1
while j > 0 and a[j] > b[i - 1]:
j -= 1
f[i] = f[i - 1] + (i - j - 1)
ans = float('inf')
for i in range(n):
cnt = i + 1 + n - bisect.bisect_left(b, a[i] + 1)
ans = min(ans, cnt + f[n] - f[i + 1])
return ans
t = int(input())
for _ in range(t):
n = int(input())
a = list(map(int, input().split()))
b = list(map(int, input().split()))
print(min_operations(a, b))
```
在修改后的代码中,我们将变量 `n` 的定义提前到函数外部,并使用 `global` 关键字声明它是全局变量。在函数中,我们使用 `n` 来初始化 `f` 数组,而在 `for` 循环中,我们使用 `n` 和 `b` 来计算 `cnt` 的值。
n,L = map(int, input().split(' ')) l = list(map(int, input().split(' '))) l.sort() sum=int(0) i=int(0) cnt=int(0) for i in range(n): if(sum<=L): sum+=l[i] cnt+=1 if(sum>L): sum-=l[i] cnt-=1 print(cnt) 时间复杂度分析
这段代码的时间复杂度为O(nlogn),其中n为输入的长度。
原因是,在第4行中,对输入的列表进行了一次排序操作,时间复杂度为O(nlogn)。之后,通过for循环遍历列表,每次判断sum是否小于等于L,如果是,则将当前数字加入sum,并将cnt加1;如果不是,则从sum中减去当前数字,并将cnt减1。由于for循环遍历了整个列表,因此时间复杂度为O(n)。因此,总的时间复杂度为O(nlogn+n),即O(nlogn)。
阅读全文
相关推荐
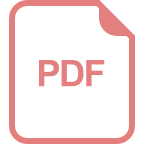
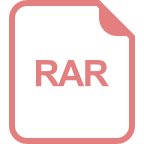













