维吉尼亚python
时间: 2023-10-18 19:31:04 浏览: 44
维吉尼亚Python是一个基于Python语言开发的密码学库,它提供了各种加密、解密和密码学相关的功能。它可以用于实现对称加密算法(如AES、DES等)、非对称加密算法(如RSA、ECC等)、哈希函数(如MD5、SHA1等)以及其他密码学操作。维吉尼亚Python库的设计目标是提供简单易用的接口,使得开发者能够方便地使用密码学算法进行数据加密和解密。如果你有更具体的问题或需要使用维吉尼亚Python库的某些功能,请告诉我详细需求。
相关问题
维吉尼亚密码python
维吉尼亚密码是一种古典密码,它利用了字母表中字母的周期性重复性质,将明文中的每个字母都通过一个固定的密码字母表中的字母进行替换,从而得到密文。下面是一个简单的 Python 实现:
```python
def vigenere_cipher(plain_text, key):
# 将明文和密钥都转换为大写字母
plain_text = plain_text.upper()
key = key.upper()
# 定义密文变量
cipher_text = ""
# 定义字母表
letters = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
# 循环遍历明文中的每个字母
for i in range(len(plain_text)):
# 获取当前字母在字母表中的索引
plain_index = letters.index(plain_text[i])
# 获取当前密钥字母在字母表中的索引
key_index = letters.index(key[i % len(key)])
# 获取替换后的字母
cipher_index = (plain_index + key_index) % 26
cipher_text += letters[cipher_index]
# 返回密文
return cipher_text
```
其中,plain_text 是明文,key 是密钥。调用该函数即可得到对应的密文。
python解密维吉尼亚
维吉尼亚加密是一种典型的分组加密方法,它对英文字母进行偏移得到新的英文字母,并且增加了密钥的长度,按分组进行加密。而维吉尼亚解密方法则是根据英文文章中26个英文字母的统计频率来进行筛选,以获得最符合的密钥。 在Python中,可以使用如下基本代码进行维吉尼亚解密:
```
def cracker(miwen, CI):
N = [0.0 * 26
CI2 = 0.0
j = 0
for i in range(len(miwen)):
if (miwen[i].isupper() or miwen[i].islower()):
j = j + 1
new_miwen = [0 * (j)
CT1 = [0 * (j)
CI1 = [0.0 * (len(new_miwen))
j = 0
for i in range(len(miwen)):
if (miwen[i].isupper() or miwen[i].islower()):
new_miwen[j = miwen[i]
j = j + 1
for i in range(1, len(new_miwen)):
for k in range(0, i):
for j in range(k, len(new_miwen), i):
N[(ord(new_miwen[j]) - 65) ** 2 = N[(ord(new_miwen[j]) - 65) ** 2 + 1
for m in range(26):
if (len(new_miwen) / i <= 20):
CI1[k = 0.0
else:
CI1[k = float(N[m * (N[m - 1)) / float((len(new_miwen) / i) * ((len(new_miwen) / i) - 1))
for n in range(len(N)):
N[n = 0.0
CI2 = CI2 + CI1[k]
CI2 = CI2 / i
CT1[i = abs(CI2 - float(CI))
CI2 = 0.0
for i in range(len(CI1)):
CI1[i = 0.0
minin = CT1 < minin):
lmin = i
minin = CT1[i]
return lmin
```
这段代码可以通过输入密文和密钥频率指数CI来解密维吉尼亚加密的文本。它会根据密文中的字母频率统计数据进行筛选,找到最符合的密钥。返回的lmin值即为最佳密钥的长度。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [维吉尼亚解密及Python实现](https://blog.csdn.net/weixin_43697025/article/details/93969299)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
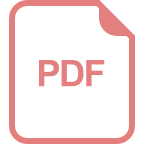












