写一段Python情感分析的代码
时间: 2024-12-24 08:38:09 浏览: 7
在Python中进行情感分析通常会使用自然语言处理库如NLTK、TextBlob或者更现代的如spaCy和transformers(基于BERT等模型)。这里是一个简单的例子,使用TextBlob库进行基础的情感极性分析:
```python
from textblob import TextBlob
def sentiment_analysis(text):
"""
使用TextBlob对给定文本进行情感分析,返回0到1之间的极性分数,其中1代表正面情绪,0代表负面情绪。
"""
blob = TextBlob(text)
# 获取情感极性,范围从-1(负面)到1(正面)
sentiment_polarity = blob.sentiment.polarity
if sentiment_polarity > 0:
return '正向'
elif sentiment_polarity < 0:
return '负向'
else:
return '中立'
# 示例使用
text = "这个电影太棒了,我喜欢看!"
print(sentiment_analysis(text)) # 输出:正向
text = "今天的天气真糟糕"
print(sentiment_analysis(text)) # 输出:负向
```
请注意,这只是一个基础的情感分析,实际应用可能需要预训练的深度学习模型进行更精确的分析。
相关问题
帮我写一个python 情感分析代码
好的,针对您提出的要求,我可以为您提供一个简单的Python情感分析代码,具体实现如下:
# 导入情感分析库
from snownlp import SnowNLP
# 输入文本信息
text = input("请输入要进行情感分析的文本:")
# 进行情感分析并输出结果
s = SnowNLP(text)
if s.sentiments > 0.5:
print("这是一段积极的文本,情感得分为:", s.sentiments)
else:
print("这是一段消极的文本,情感得分为:", s.sentiments)
这个代码使用SnowNLP库进行情感分析,首先引入库,然后通过用户输入的文本信息进行情感分析,最后输出结果。如果情感得分大于0.5,则判定为积极的文本;否则,判定为消极的文本。
python用知网情感分析词典写一段情感分类的代码
可以使用Python中的NLTK(自然语言处理工具包)和知网情感分析词典来进行情感分类。
以下是一个使用知网情感分析词典进行情感分类的Python代码示例:
首先,导入所需的库和词典文件:
```
import jieba
from nltk.corpus import CategorizedPlaintextCorpusReader
from nltk.tokenize import word_tokenize
# 加载知网情感分析词典
pos_dict_file = 'path/to/pos_dict.txt'
neg_dict_file = 'path/to/neg_dict.txt'
pos_dict = set([line.strip() for line in open(pos_dict_file, encoding='utf-8') if line.strip()])
neg_dict = set([line.strip() for line in open(neg_dict_file, encoding='utf-8') if line.strip()])
```
接下来,定义一个函数,用于对输入的文本进行情感分类:
```
def classify_sentiment(text):
# 分词
words = jieba.cut(text)
# 计算文本中正向情感词和负向情感词的数量
pos_count = sum([1 for word in words if word in pos_dict])
neg_count = sum([1 for word in words if word in neg_dict])
# 根据正向情感词和负向情感词的数量来判断情感倾向
if pos_count > neg_count:
return 'positive'
elif pos_count < neg_count:
return 'negative'
else:
return 'neutral'
```
最后,对输入的文本进行情感分类:
```
text = '这个电影真的很棒,非常值得一看!'
sentiment = classify_sentiment(text)
print(sentiment) # 输出:positive
```
这段代码使用了中文分词工具jieba对输入的文本进行分词,然后遍历分词后的单词列表,计算其中正向情感词和负向情感词的数量,最后根据数量比较来判断情感倾向。如果正向情感词的数量多于负向情感词的数量,则将情感分类为“positive”,如果负向情感词的数量多于正向情感词的数量,则将情感分类为“negative”,如果两者数量相等,则将情感分类为“neutral”。
阅读全文
相关推荐
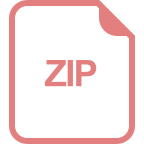
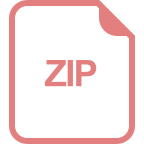
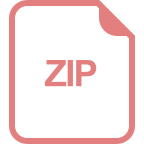













