springboot实现web文件上传下载
时间: 2023-12-21 16:03:28 浏览: 31
要在Spring Boot中实现文件上传和下载功能,可以按照以下步骤进行操作:
1. 配置文件上传的属性
在application.properties(或application.yml)文件中,添加以下配置来指定文件上传的临时存储目录和最大文件大小限制:
```
spring.servlet.multipart.enabled=true
spring.servlet.multipart.file-size-threshold=2KB
spring.servlet.multipart.max-file-size=200MB
spring.servlet.multipart.max-request-size=215MB
```
2. 创建文件上传接口
创建一个Controller来处理文件上传请求,例如:
```java
@RestController
public class FileUploadController {
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file) {
// 处理文件上传逻辑
// 返回上传成功信息
}
}
```
3. 创建文件下载接口
创建一个Controller来处理文件下载请求,例如:
```java
@RestController
public class FileDownloadController {
@GetMapping("/download")
public ResponseEntity<Resource> downloadFile() {
// 处理文件下载逻辑
// 返回文件资源及相关响应头信息
}
}
```
4. 处理文件上传逻辑
在文件上传接口中,可以使用`MultipartFile`来处理文件上传。可以使用`transferTo()`方法将文件保存到指定目录,例如:
```java
String filePath = "/path/to/save/file";
File destFile = new File(filePath);
file.transferTo(destFile);
```
5. 处理文件下载逻辑
在文件下载接口中,可以使用`ResponseEntity`来返回文件资源,并设置相关的响应头信息,例如:
```java
String filePath = "/path/to/file";
File file = new File(filePath);
FileSystemResource resource = new FileSystemResource(file);
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=" + file.getName())
.contentLength(file.length())
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.body(resource);
```
请注意,上述代码中的文件路径和保存目录需要根据实际情况进行修改。
希望这些步骤能帮助你实现Spring Boot中的文件上传和下载功能!
相关推荐
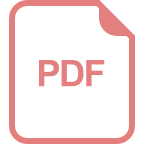
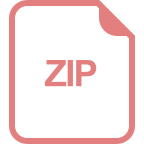








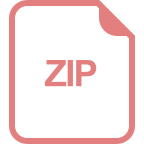
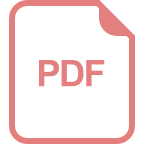
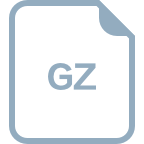
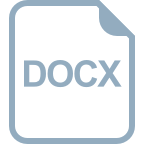
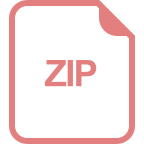