SDL库中点算法绘制椭圆,请完成这个函数void drawEllipse(int cx, int cy, int a, int b, unsigned int color)
时间: 2024-09-21 21:12:38 浏览: 45
在Simple DirectMedia Layer (SDL) 库中,虽然SDL本身主要是用于图形窗口和输入处理,它并没有直接提供内置的函数来绘制精确的椭圆。不过,你可以通过一些数学计算和循环来模拟椭圆的绘制。下面是一个简单的示例,展示如何在一个基本的框架内手动实现这个功能。请注意,这并不是SDL官方提供的API,而是利用了像素级别的操作:
```cpp
#include <math.h>
// 假设有一个结构体保存像素颜色信息
struct PixelColor {
Uint32 color;
};
// 模拟一个画像素的辅助函数
void setPixel(PixelColor* pixels, int x, int y, unsigned int color) {
pixels[x + y * width] = {color};
}
// 绘制椭圆的基本函数
void drawEllipse(int cx, int cy, int a, int b, PixelColor* pixels, int width, int height) {
int x, y;
// 确保a <= b(因为椭圆的主轴长度)
if (a > b) {
std::swap(a, b);
}
for (x = -a; x <= a; ++x) {
double t = atan2(x, b) / M_PI; // 将x转换到[0, 2π]区间内的角度
// 画水平线段
y = round(cy + a * sin(t));
if (y >= 0 && y < height) {
setPixel(pixels, cx + x, y, color);
}
// 画垂直线段
y = round(cy - a * cos(t));
if (y >= 0 && y < height) {
setPixel(pixels, cx + x, y, color);
}
}
}
// 使用函数
int main() {
// 初始化 SDL 等...
PixelColor screenBuffer[width * height]; // 假设screenBuffer是你的像素数组
drawEllipse(cx, cy, a, b, screenBuffer, width, height);
// 更新屏幕...
return 0;
}
```
阅读全文
相关推荐
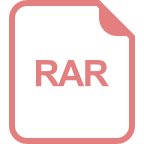
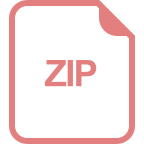
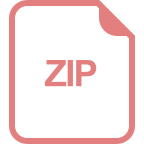
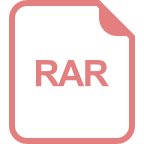
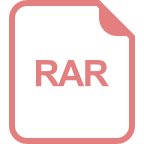
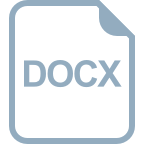
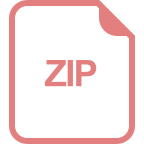
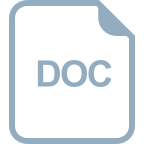
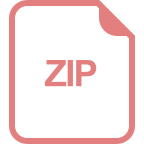
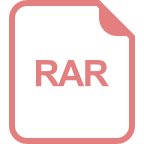
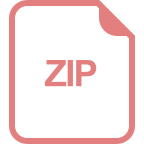
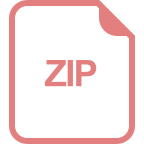
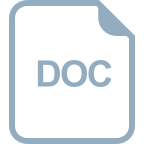

