六:类和对象 1、 创建球类。属性包括球的半径和颜色,计算球体的面积和体积、 2、 设计一个Person(人)类,包括姓名,年龄,和血型属性,编写构造方法,用于初始化每个人的具体属性,编写detail方法用于输出每个实例具体值。 3、 设计一个Animal(动物)类,包括颜色属性和叫方法,再设计一个Fish类(鱼)类。包括尾巴和颜色两个属性,以及叫法。要求:Fish类继承自Animal类,重写构造方法和叫方法 七: 文件与异常 1、创建文件,名称为“english_song.txt”,将歌词按行输入 2、将文件重命名为“my heart will go on.txt” 3、对文件创建副本为“back-up.txt” 4、关闭文件
时间: 2024-02-23 08:58:25 浏览: 74
六:
1. 球类的代码实现:
```python
import math
class Ball:
def __init__(self, radius, color):
self.radius = radius
self.color = color
def area(self):
return 4*math.pi*self.radius**2
def volume(self):
return 4/3*math.pi*self.radius**3
```
2. Person类的代码实现:
```python
class Person:
def __init__(self, name, age, blood_type):
self.name = name
self.age = age
self.blood_type = blood_type
def detail(self):
print(f"姓名:{self.name},年龄:{self.age},血型:{self.blood_type}")
```
3. Animal类和Fish类的代码实现:
```python
class Animal:
def __init__(self, color):
self.color = color
def call(self):
print("动物在叫")
class Fish(Animal):
def __init__(self, color, tail):
super().__init__(color)
self.tail = tail
def call(self):
print("鱼在唱歌")
```
七:
1. 创建文件并按行输入歌词的代码实现:
```python
with open("english_song.txt", "w") as file:
file.write("You are my fire, the one desire\n")
file.write("Believe when I say, I want it that way\n")
file.write("But we are two worlds apart\n")
file.write("Can't reach to your heart\n")
```
2. 将文件重命名的代码实现:
```python
import os
os.rename("english_song.txt", "my heart will go on.txt")
```
3. 创建文件副本的代码实现:
```python
import shutil
shutil.copy("my heart will go on.txt", "back-up.txt")
```
4. 关闭文件的代码实现:
```python
file.close()
```
相关推荐
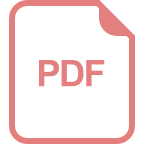










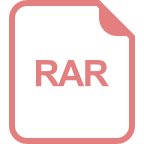
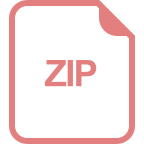