利用内核模块在/proc文件系统目录下创建proc_example目录,并在该目录下创建三个普通文件(foo,bar,jiffies)和一个文件链接(jiffies_too)代码
时间: 2024-03-11 14:50:01 浏览: 34
下面是一个示例内核模块代码,用于在/proc文件系统目录下创建proc_example目录,并在该目录下创建三个普通文件(foo,bar,jiffies)和一个文件链接(jiffies_too):
```c
#include <linux/init.h>
#include <linux/module.h>
#include <linux/proc_fs.h>
#include <linux/uaccess.h>
#include <linux/jiffies.h>
#define BUF_SIZE 100
static struct proc_dir_entry *proc_example_dir;
static struct proc_dir_entry *foo_file;
static struct proc_dir_entry *bar_file;
static struct proc_dir_entry *jiffies_file;
static struct proc_dir_entry *jiffies_too_file;
static char buf[BUF_SIZE];
static int len = 0;
static ssize_t foo_read(struct file *file, char __user *ubuf, size_t count, loff_t *ppos)
{
if (*ppos > 0 || count < len)
return 0;
if (copy_to_user(ubuf, buf, len))
return -EFAULT;
*ppos = len;
return len;
}
static ssize_t foo_write(struct file *file, const char __user *ubuf, size_t count, loff_t *ppos)
{
if (count > BUF_SIZE)
return -EINVAL;
if (copy_from_user(buf, ubuf, count))
return -EFAULT;
len = count;
return len;
}
static struct file_operations foo_fops = {
.owner = THIS_MODULE,
.read = foo_read,
.write = foo_write,
};
static int __init proc_example_init(void)
{
proc_example_dir = proc_mkdir("proc_example", NULL);
if (proc_example_dir == NULL) {
printk(KERN_ALERT "Error: Could not create proc_example directory\n");
return -ENOMEM;
}
foo_file = proc_create("foo", 0666, proc_example_dir, &foo_fops);
if (foo_file == NULL) {
printk(KERN_ALERT "Error: Could not create foo file\n");
remove_proc_entry("proc_example", NULL);
return -ENOMEM;
}
bar_file = proc_create("bar", 0666, proc_example_dir, NULL);
if (bar_file == NULL) {
printk(KERN_ALERT "Error: Could not create bar file\n");
remove_proc_entry("foo", proc_example_dir);
remove_proc_entry("proc_example", NULL);
return -ENOMEM;
}
jiffies_file = proc_create("jiffies", 0444, proc_example_dir, NULL);
if (jiffies_file == NULL) {
printk(KERN_ALERT "Error: Could not create jiffies file\n");
remove_proc_entry("bar", proc_example_dir);
remove_proc_entry("foo", proc_example_dir);
remove_proc_entry("proc_example", NULL);
return -ENOMEM;
}
jiffies_too_file = proc_symlink("jiffies_too", proc_example_dir, "jiffies");
if (jiffies_too_file == NULL) {
printk(KERN_ALERT "Error: Could not create jiffies_too symlink\n");
remove_proc_entry("jiffies", proc_example_dir);
remove_proc_entry("bar", proc_example_dir);
remove_proc_entry("foo", proc_example_dir);
remove_proc_entry("proc_example", NULL);
return -ENOMEM;
}
len = sprintf(buf, "Hello World\n");
return 0;
}
static void __exit proc_example_exit(void)
{
remove_proc_entry("jiffies_too", proc_example_dir);
remove_proc_entry("jiffies", proc_example_dir);
remove_proc_entry("bar", proc_example_dir);
remove_proc_entry("foo", proc_example_dir);
remove_proc_entry("proc_example", NULL);
}
MODULE_LICENSE("GPL");
MODULE_DESCRIPTION("Example proc file system module");
MODULE_AUTHOR("Your Name");
module_init(proc_example_init);
module_exit(proc_example_exit);
```
这段代码创建了一个名为proc_example的目录,其中包含三个普通文件(foo,bar,jiffies)和一个文件链接(jiffies_too)。foo文件具有读/写权限,并使用读和写操作将数据存储在buf缓冲区中。bar文件只有读权限,因此不需要指定操作。jiffies文件只有读权限,并在读取时返回当前的jiffies值。jiffies_too文件是一个链接,指向jiffies文件。
请注意,此代码仅为示例,可能需要进行修改才能在您的系统上运行,例如更改作者名称和模块描述,或更改文件和目录的权限。
相关推荐
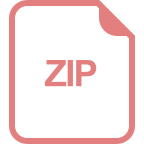
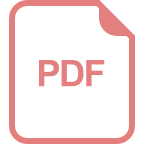
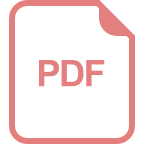














