Leaky ReLU代码
时间: 2023-07-09 15:53:32 浏览: 143
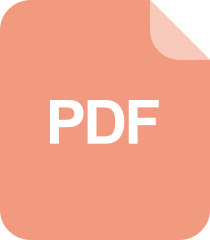
在Tensorflow中实现leakyRelu操作详解(高效)
Leaky ReLU是ReLU的一个变种,可以在输入小于0的时候,给出一个小的负斜率,以解决ReLU可能出现的“神经元死亡”问题。以下是Python代码实现:
```python
import tensorflow as tf
def leaky_relu(x, alpha=0.2):
return tf.maximum(alpha * x, x)
```
其中,alpha为斜率,通常取0.01或0.2。在使用时,将该函数作为激活函数传入神经网络中的某一层即可。例如:
```python
model.add(layers.Dense(64, activation=leaky_relu))
```
阅读全文
相关推荐
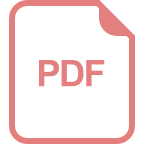
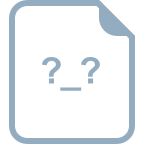















