实现图像增强的直方图均衡 c++
时间: 2023-05-29 12:04:36 浏览: 104
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define WIDTH 512
#define HEIGHT 512
#define MAX_PIXEL_VALUE 255
void histogram_equalization(int *histogram, int *mapping);
int main()
{
FILE *input_file, *output_file;
unsigned char input_image[WIDTH][HEIGHT], output_image[WIDTH][HEIGHT];
int histogram[MAX_PIXEL_VALUE + 1] = {0};
int mapping[MAX_PIXEL_VALUE + 1] = {0};
int i, j;
// Open input file
if ((input_file = fopen("input_image.raw", "rb")) == NULL)
{
printf("Error: Can't open input file\n");
return 1;
}
// Read input image
fread(input_image, sizeof(unsigned char), WIDTH * HEIGHT, input_file);
// Calculate histogram
for (i = 0; i < WIDTH; i++)
{
for (j = 0; j < HEIGHT; j++)
{
histogram[input_image[i][j]]++;
}
}
// Equalize histogram
histogram_equalization(histogram, mapping);
// Apply mapping to input image
for (i = 0; i < WIDTH; i++)
{
for (j = 0; j < HEIGHT; j++)
{
output_image[i][j] = mapping[input_image[i][j]];
}
}
// Open output file
if ((output_file = fopen("output_image.raw", "wb")) == NULL)
{
printf("Error: Can't open output file\n");
return 1;
}
// Write output image
fwrite(output_image, sizeof(unsigned char), WIDTH * HEIGHT, output_file);
// Close files
fclose(input_file);
fclose(output_file);
return 0;
}
void histogram_equalization(int *histogram, int *mapping)
{
int cdf[MAX_PIXEL_VALUE + 1] = {0};
int i, j;
float total_pixels = WIDTH * HEIGHT;
// Calculate cumulative distribution function
cdf[0] = histogram[0];
for (i = 1; i <= MAX_PIXEL_VALUE; i++)
{
cdf[i] = cdf[i - 1] + histogram[i];
}
// Normalize cdf values
for (i = 0; i <= MAX_PIXEL_VALUE; i++)
{
cdf[i] = round((cdf[i] / total_pixels) * MAX_PIXEL_VALUE);
}
// Create mapping
for (i = 0; i <= MAX_PIXEL_VALUE; i++)
{
mapping[i] = cdf[i];
}
}
阅读全文
相关推荐
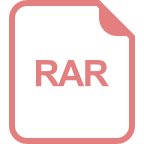
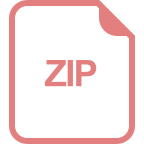
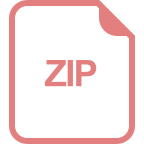
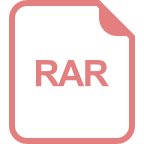


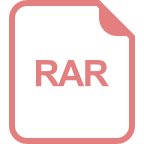
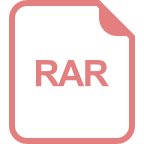
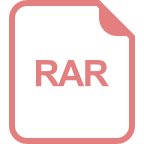
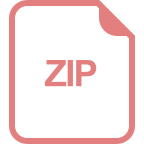
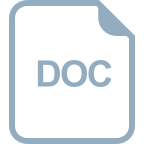
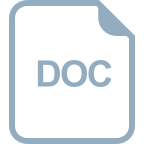
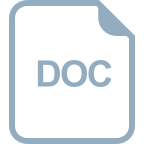
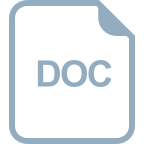
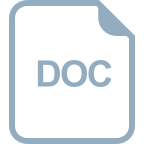