vue怎么使用onclick选中当前vue页面方法
时间: 2023-08-29 11:06:08 浏览: 49
在Vue中,可以使用`@click`或`v-on:click`指令来绑定点击事件。如果要在点击事件中获取当前Vue实例,可以使用`this`关键字。以下是一个示例:
```html
<template>
<div @click="handleClick">
Click me!
</div>
</template>
<script>
export default {
methods: {
handleClick() {
console.log('当前Vue实例:', this);
// 在这里可以使用this来调用当前Vue实例的方法或访问其数据
}
}
}
</script>
```
在上面的示例中,当点击`<div>`元素时,会触发`handleClick`方法。在该方法中,使用`this`关键字来获取当前Vue实例,并可以在方法中使用该实例来访问其数据或调用其方法。
相关问题
vue3 antd tabClick的用法
好的,我理解了。关于你的问题,Vue3 Antd中的Tab组件具有一个onClick属性,你可以将其绑定到一个methods方法中。当Tab被点击时,该方法将被调用。以下是一个使用Vue3 Antd Tab组件的示例代码:
```
<template>
<a-tabs v-model:selectedKey="selectedKey">
<a-tab v-for="(tab, index) in tabs" :key="tab.key" :tab="tab.tab">
<span slot="tab">{{tab.title}}</span>
<p>{{tab.content}}</p>
</a-tab>
</a-tabs>
</template>
<script>
import { ref } from 'vue';
import { Tabs } from 'ant-design-vue';
export default {
components: {
'a-tabs': Tabs,
'a-tab': Tabs.TabPane
},
setup() {
const selectedKey = ref('1');
const tabs = [
{
key: '1',
title: 'Tab1',
content: 'This is Tab1 content.',
tab: {
onClick: () => {
console.log('Tab1 is clicked');
}
}
},
{
key: '2',
title: 'Tab2',
content: 'This is Tab2 content.',
tab: {
onClick: () => {
console.log('Tab2 is clicked');
}
}
}
];
return {
selectedKey,
tabs
};
}
};
</script>
```
在上面的代码中,我们使用了Vue3中的ref来创建了一个selectedKey变量和tabs数组变量。通过selectedKey变量来控制当前选中的Tab项,通过tabs数组变量来定义Tab的相关信息,包括标题、内容和点击回调函数。 在a-tab组件中,我们使用了Vue3中的v-for指令来遍历tabs数组,并循环渲染出每个Tab项。 在Tab中,我们为onClick属性绑定了一个方法,当Tab被点击时该方法将被调用。
希望这能够解决你的问题,如果你还有其他问题,请告诉我。
写一个vue日期时间选择器
好的,以下是一个基于 Element UI 的 Vue.js 日期时间选择器示例代码。
前端代码:
```
<template>
<div>
<el-date-picker
v-model="date"
type="datetime"
placeholder="选择日期时间"
:picker-options="pickerOptions"
:default-time="['00:00:00']"
:value-format="'yyyy-MM-dd HH:mm:ss'"
@change="handleChange">
</el-date-picker>
</div>
</template>
<script>
export default {
data() {
return {
date: '',
pickerOptions: {
shortcuts: [{
text: '今天',
onClick(picker) {
picker.$emit('pick', new Date());
}
}, {
text: '昨天',
onClick(picker) {
const date = new Date();
date.setTime(date.getTime() - 24 * 60 * 60 * 1000);
picker.$emit('pick', date);
}
}, {
text: '一周前',
onClick(picker) {
const date = new Date();
date.setTime(date.getTime() - 7 * 24 * 60 * 60 * 1000);
picker.$emit('pick', date);
}
}]
}
};
},
methods: {
handleChange(value) {
console.log(value);
}
}
};
</script>
```
其中,`el-date-picker` 是一个 Element UI 中的日期时间选择器组件。`v-model` 用于双向绑定选中的日期时间,`type` 用于指定选择器类型为日期时间,`placeholder` 用于设置占位符文本,`picker-options` 用于设置选择器的选项,`default-time` 用于设置默认时间,`value-format` 用于设置日期时间格式,`@change` 用于监听日期时间变化事件。
后端代码:
由于日期时间选择器是前端组件,所以不需要后端代码。如果您需要在后端处理日期时间数据,可以使用 Java 的日期时间 API 或者其他语言相应的 API 进行处理。
相关推荐
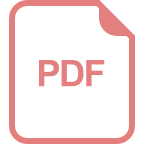
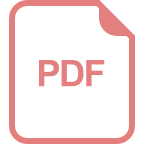
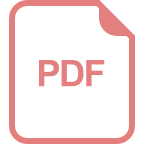












