帮我写一段目标检测函数
时间: 2023-10-18 18:14:13 浏览: 46
好的,以下是一个使用 TensorFlow Object Detection API 实现目标检测的函数示例:
```python
import tensorflow as tf
from object_detection.utils import ops as utils_ops
from object_detection.utils import label_map_util
from object_detection.utils import visualization_utils as vis_util
def detect_objects(image_path, model_path, label_path, confidence_threshold=0.5):
# Load image
image = tf.io.read_file(image_path)
image = tf.image.decode_jpeg(image, channels=3)
image = tf.expand_dims(image, axis=0)
# Load model
detection_model = tf.saved_model.load(model_path)
detection_fn = detection_model.signatures['serving_default']
# Load label map
label_map = label_map_util.load_labelmap(label_path)
categories = label_map_util.convert_label_map_to_categories(label_map, max_num_classes=90, use_display_name=True)
category_index = label_map_util.create_category_index(categories)
# Run inference
detections = detection_fn(image)
# Process output
num_detections = int(detections.pop('num_detections'))
detections = {key: value[0, :num_detections].numpy() for key, value in detections.items()}
detections['num_detections'] = num_detections
detections['detection_classes'] = detections['detection_classes'].astype(np.int64)
image_np_with_detections = image.numpy().squeeze()
# Visualize results
vis_util.visualize_boxes_and_labels_on_image_array(
image_np_with_detections,
detections['detection_boxes'],
detections['detection_classes'],
detections['detection_scores'],
category_index,
use_normalized_coordinates=True,
max_boxes_to_draw=200,
min_score_thresh=confidence_threshold,
agnostic_mode=False)
# Return image with detections
return image_np_with_detections
```
该函数需要传入以下参数:
- `image_path`: 待检测的图像路径
- `model_path`: TensorFlow Object Detection API 模型路径
- `label_path`: 标签映射文件路径
- `confidence_threshold`: 置信度阈值,低于该阈值的检测结果将被过滤掉,默认为 0.5
函数内部使用 TensorFlow 加载图像、模型和标签映射文件,并通过 Object Detection API 进行推理和结果处理。最后,将可视化结果返回。
相关推荐
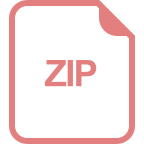
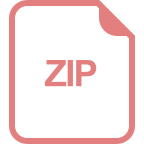














