用python写一段目标识别的代码
时间: 2023-09-17 21:05:43 浏览: 68
### 回答1:
答:我们可以使用Python来编写目标识别代码,我们先需要从图像中提取出特征,然后构建一个分类器,用它来对图像中的目标进行识别。下面是一段Python代码,用来进行目标识别: # Extract features from the image
features = extract_features(image) # Build the classifier
clf = build_classifier(features) # Use the classifier to predict the target
prediction = clf.predict(features)
### 回答2:
以下是一段使用Python编写的目标识别代码:
```python
import cv2
import numpy as np
def target_recognition(image_path, target_path):
# 加载图像和目标
image = cv2.imread(image_path)
target = cv2.imread(target_path)
# 创建ORB特征检测器
orb = cv2.ORB_create()
# 使用ORB检测图像和目标的关键点和描述符
kp_image, des_image = orb.detectAndCompute(image, None)
kp_target, des_target = orb.detectAndCompute(target, None)
# 使用暴力匹配器进行特征点匹配
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
matches = bf.match(des_image, des_target)
matches = sorted(matches, key = lambda x:x.distance)
# 绘制匹配结果
result = cv2.drawMatches(image, kp_image, target, kp_target, matches[:10], None, flags=2)
# 展示结果图像
cv2.imshow("Target Recognition", result)
cv2.waitKey(0)
cv2.destroyAllWindows()
# 调用函数进行目标识别
target_recognition("image.jpg", "target.jpg")
```
该段代码使用了OpenCV库中的ORB特征检测器和暴力匹配器来进行目标识别。首先,通过`cv2.imread()`函数加载图像和目标。然后,创建一个ORB特征检测器,并使用它来检测图像和目标的关键点和描述符。随后,使用暴力匹配器进行特征点匹配,并将结果绘制在结果图像上。最后,使用`cv2.imshow()`函数展示结果图像,并通过`cv2.waitKey(0)`等待用户按下任意键来关闭展示窗口。
请确保将`image.jpg`和`target.jpg`替换为实际的图像和目标路径。请注意,该代码仅实现了最基本的目标识别功能,对于不同场景和更复杂的目标,可能需要使用更高级的算法和技术来提高识别准确度。
### 回答3:
目标识别在计算机视觉领域中是一个重要的应用,Python提供了许多强大的图像处理库,如OpenCV和TensorFlow,可以用于实现目标识别的代码。
以下是一段使用OpenCV库实现目标识别的Python代码示例:
```python
import cv2
def target_detection(image_path, target_image_path):
# 加载图像和目标图像
image = cv2.imread(image_path)
target_image = cv2.imread(target_image_path)
# 创建SURF特征检测器
surf = cv2.xfeatures2d.SURF_create()
# 在图像中检测关键点和描述符
kp1, des1 = surf.detectAndCompute(target_image, None)
kp2, des2 = surf.detectAndCompute(image, None)
# 创建FLANN匹配器
matcher = cv2.FlannBasedMatcher()
# 使用knnMatch方法进行特征匹配
matches = matcher.knnMatch(des1, des2, k=2)
# 过滤匹配结果
good_matches = []
for m, n in matches:
if m.distance < 0.7 * n.distance:
good_matches.append(m)
# 绘制匹配结果
result = cv2.drawMatches(target_image, kp1, image, kp2, good_matches, None, flags=2)
# 显示结果图像
cv2.imshow("Target Detection", result)
cv2.waitKey(0)
cv2.destroyAllWindows()
# 调用目标检测函数
target_detection("image.jpg", "target_image.jpg")
```
这段代码通过SURF算法检测图像中的关键点和描述符,并利用FLANN匹配器进行特征匹配,最后绘制出匹配结果图像并显示。
需要注意的是,目标识别是一个复杂的问题,并且有很多不同的方法和算法可以用于实现。以上代码只是其中一种实现方式,具体的实现根据目标识别的需求和数据集的特征可能会有所不同。
相关推荐
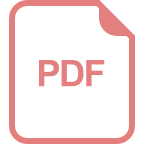
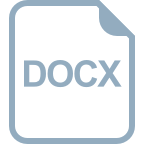
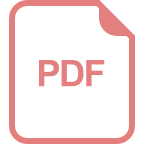














