python 如何查看tensor形状
时间: 2023-07-26 07:17:50 浏览: 50
在Python中,可以使用PyTorch或TensorFlow等框架创建tensor。查看tensor形状的方法也略有不同:
1. PyTorch中,可以使用`.shape`属性来查看tensor的形状,例如:
```
import torch
x = torch.randn(3, 4, 5)
print(x.shape)
```
输出结果为`(3, 4, 5)`,表示这是一个3维的tensor,第一维大小为3,第二维大小为4,第三维大小为5。
2. TensorFlow中,可以使用`.shape`属性或`tf.shape()`函数来查看tensor的形状,例如:
```
import tensorflow as tf
x = tf.random.normal([3, 4, 5])
print(x.shape)
print(tf.shape(x))
```
输出结果为`(3, 4, 5)`和`(3, 4, 5)`,两种方法都可以查看tensor的形状。
注意,在PyTorch和TensorFlow中,tensor的形状通常是一个tuple或tensor对象。
相关问题
python 求tensor最大值
要在 Python 中求一个 tensor 的最大值,你可以使用 PyTorch 或 TensorFlow 这样的库。下面是使用 PyTorch 的示例代码:
```python
import torch
# 创建一个 tensor
tensor = torch.tensor([1, 2, 3, 4, 5])
# 求最大值
max_value = torch.max(tensor)
print(max_value)
```
使用 TensorFlow 的示例代码如下:
```python
import tensorflow as tf
# 创建一个 tensor
tensor = tf.constant([1, 2, 3, 4, 5])
# 求最大值
max_value = tf.reduce_max(tensor)
print(max_value)
```
这些代码将打印出 tensor 中的最大值。你可以根据自己的需求调整 tensor 的形状和数据类型。
Python中tensor的具体用法
在Python中,使用Tensor的具体用法可以根据不同的库和框架而有所不同。以下是一些常见的Python库和框架中Tensor的具体用法示例:
1. NumPy库:
- 创建Tensor:使用`numpy.array()`函数可以创建一个NumPy的ndarray对象,它可以表示一个多维数组。例如:`import numpy as np; tensor = np.array([[1, 2, 3], [4, 5, 6]])`
- 数学运算:NumPy提供了丰富的数学运算函数和方法,可以对Tensor进行各种数学运算。例如:`np.sum(tensor)`可以计算Tensor元素的总和。
- 索引和切片:可以使用索引和切片操作来访问和修改Tensor中的元素。例如:`tensor[0, 1]`可以访问第一行第二列的元素。
- 形状操作:使用`ndarray.shape`属性可以获取Tensor的形状。例如:`tensor.shape`可以获取Tensor的维度信息。
2. TensorFlow库:
- 创建Tensor:使用`tf.constant()`函数可以创建一个TensorFlow的tf.Tensor对象。例如:`import tensorflow as tf; tensor = tf.constant([[1, 2, 3], [4, 5, 6]])`
- 运算操作:TensorFlow提供了各种张量运算操作,如加法、乘法、矩阵乘法等。例如:`tf.add(tensor1, tensor2)`可以对两个Tensor进行加法操作。
- 张量变换:使用`tf.reshape()`函数可以改变Tensor的形状。例如:`tf.reshape(tensor, [3, 2])`可以将一个2x3的Tensor转换为一个3x2的Tensor。
- GPU加速:TensorFlow可以利用GPU进行计算,可以使用`tf.device()`函数将Tensor放置在GPU上进行计算。
3. PyTorch库:
- 创建Tensor:使用`torch.Tensor()`函数可以创建一个PyTorch的torch.Tensor对象。例如:`import torch; tensor = torch.Tensor([[1, 2, 3], [4, 5, 6]])`
- 运算操作:PyTorch提供了各种张量运算操作,如加法、乘法、矩阵乘法等。例如:`torch.add(tensor1, tensor2)`可以对两个Tensor进行加法操作。
- 自动求导:PyTorch提供了自动求导功能,可以通过设置`requires_grad=True`来追踪Tensor的梯度信息,并进行反向传播。例如:`tensor.requires_grad=True`
- 模型训练:PyTorch可以方便地构建和训练神经网络模型。可以使用`torch.nn.Module`类定义模型结构,使用`torch.optim`模块定义优化器,使用`torch.nn`模块定义各种层和激活函数。
以上是一些常见的Python库和框架中Tensor的具体用法示例,具体的用法还可以根据具体需求和场景进行进一步学习和探索。
相关推荐
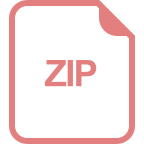
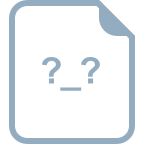
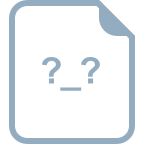












