函数指针作为结构体成员C语言
时间: 2024-09-25 09:06:29 浏览: 43
在C语言中,函数指针作为一种特殊的指针,可以用于存储函数的地址。当它作为结构体的一个成员时,我们可以创建这样的结构来封装功能,或者提供灵活性,允许我们通过这个结构来调用不同的函数。这种设计通常出现在回调函数、事件处理或需要动态绑定操作的场景中。
例如:
```c
typedef void (*func_ptr)(int); // 定义了一个指向void型函数,接受一个整数参数的指针
struct my_struct {
int data;
func_ptr callback; // 将函数指针作为结构体成员
};
void function_to_call(int arg) {
printf("Received argument: %d\n", arg);
}
int main() {
struct my_struct s = {10, &function_to_call}; // 初始化时传入函数的地址
s.callback(5); // 调用函数时,通过结构体的callback引用
return 0;
}
```
在这个例子中,`&function_to_call`是一个函数指针,当我们调用`s.callback(5)`时,实际上是调用了`function_to_call`并传递了5作为参数。
相关问题
结构体指针函数 形参为结构体指针 c语言程序例子
结构体指针函数是指函数的形参为结构体指针。通过使用结构体指针作为函数的形参,可以在函数内部对结构体进行修改,从而实现对结构体的操作。下面是一个C语言程序的例子,演示了结构体指针函数的用法:
```c
#include <stdio.h>
// 定义一个结构体
struct Student {
char name[20];
int age;
};
// 结构体指针函数,修改结构体的值
void modifyStudent(struct Student *ptr) {
// 修改结构体的值
strcpy(ptr->name, "John");
ptr->age = 20;
}
int main() {
// 定义一个结构体变量
struct Student s;
// 调用结构体指针函数,传递结构体指针作为参数
modifyStudent(&s);
// 输出修改后的结构体的值
printf("Name: %s\n", s.name); // 输出:Name: John
printf("Age: %d\n", s.age); // 输出:Age: 20
return 0;
}
```
在上面的例子中,我们定义了一个结构体`Student`,包含了姓名和年龄两个成员变量。然后我们定义了一个结构体指针函数`modifyStudent`,该函数的形参为结构体指针`ptr`。在函数内部,我们通过指针修改了结构体的值。最后,在`main`函数中,我们创建了一个结构体变量`s`,并将其地址传递给`modifyStudent`函数进行修改。最终,我们输出了修改后的结构体的值。
c语言结构体成员函数
C语言的结构体成员函数是通过函数指针实现的。在C语言中,结构体是不允许包含成员函数的,但可以通过定义函数指针,并将其指向相应的函数,来实现类似的功能。可以将函数指针作为结构体的成员,并在结构体变量中通过函数指针来调用相应的函数。
以下是一个示例代码,展示了如何在C语言的结构体中定义和使用成员函数:
```
/* 定义两个子函数 */
void function1(int a, int b) {
printf("a b的值为:%d\r\n", (a + b));
}
int function2(int c) {
printf("c的值为:%d\r\n", c);
return c;
}
/* 结构体类型的定义 */
struct Example{
int a;
int b;
void (*function1)(int a, int b);
int (*function2)(int c);
};
/* 结构体变量的定义 */
struct Example example = {10, 10, function1, function2}; // 指定结构体中各成员的具体意义
/* 调用成员函数 */
example.function1(1, 2);
example.function2(100);
```
在上述示例中,我们定义了一个名为Example的结构体,它包含了两个成员变量a和b,以及两个函数指针function1和function2。通过指定函数指针指向相应的函数,并在结构体变量example中调用这些成员函数,实现了结构体的成员函数功能。
阅读全文
相关推荐



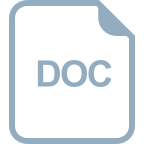
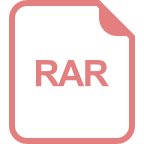










