python 字符中replace 用 \取代\\
时间: 2024-04-18 17:22:57 浏览: 21
在Python中,字符串的replace()方法可以用于替换字符串中的指定子串。当需要将一个反斜杠(\)替换为两个反斜杠(\\)时,可以使用以下代码:
```python
string = "python 字符中replace 用 \\ 取代\\\\"
new_string = string.replace("\\", "\\\\")
print(new_string)
```
输出结果为:"python 字符中replace 用 \\\\ 取代\\\\\\\\"
在replace()方法中,第一个参数是要被替换的子串,第二个参数是替换后的子串。由于反斜杠是一个转义字符,所以在替换时需要使用两个反斜杠来表示一个反斜杠。
相关问题
python中字符串replace
在Python中,字符串的`replace()`方法用于将指定的子字符串替换为新的字符串。它的语法如下:
```python
string.replace(old, new, count)
```
其中,`string`是要进行替换操作的字符串,`old`是要被替换的子字符串,`new`是替换后的新字符串,`count`是可选参数,表示替换的次数。
`replace()`方法会返回一个新的字符串,原始字符串不会被修改。如果不指定`count`参数,则会替换所有匹配的子字符串;如果指定了`count`参数,则只会替换前`count`个匹配的子字符串。
下面是一个示例:
```python
string = "Hello, World!"
new_string = string.replace("Hello", "Hi")
print(new_string) # 输出:Hi, World!
```
python 字符串replace
Python 的字符串 replace() 方法用于将字符串中的某个部分替换成另一个字符串。
语法:
str.replace(old, new[, count])
参数:
old - 要被替换的字符串
new - 替换成的字符串
count - 替换的次数,默认替换所有
示例:
string = "Hello World"
print(string.replace("World", "Python"))
#将"World" 替换成 "Python"
输出:
Hello Python
相关推荐
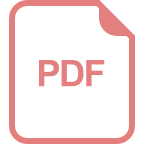
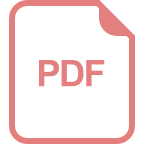
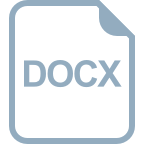












