@Test public void testLabelOrder() throws Exception { ManInfo recManInfo = new ManInfo(); recManInfo.setName("张三"); recManInfo.setMobile("15999566666"); recManInfo.setPrintAddr("广东省深圳市南山区科技南十二路"); ManInfo sendManInfo = new ManInfo(); sendManInfo.setName("李四"); sendManInfo.setMobile("15999566666"); sendManInfo.setPrintAddr("北京市海淀区xxx路"); OrderReq orderReq = new OrderReq(); orderReq.setKuaidicom(CompanyConstant.ZJS); orderReq.setCount(1); orderReq.setSiid(siid); orderReq.setTempId("60f6c17c7c223700131d8bc3"); orderReq.setSendMan(sendManInfo); orderReq.setRecMan(recManInfo); orderReq.setPrintType(PrintType.CLOUD); String param = new Gson().toJson(orderReq); String t = System.currentTimeMillis() + ""; PrintReq printReq = new PrintReq(); printReq.setT(t); printReq.setKey(key); printReq.setSign(SignUtils.printSign(param,t,key,secret)); printReq.setMethod(ApiInfoConstant.ORDER); printReq.setParam(param); IBaseClient baseClient = new LabelV2(); System.out.println(baseClient.execute(printReq)); }
时间: 2024-04-29 20:20:25 浏览: 77
这段代码是用于测试一个快递公司的订单打印功能的。首先,定义了两个人的信息,一个是寄件人(sendManInfo),一个是收件人(recManInfo)。然后,定义了一个OrderReq类的实例orderReq,包含了快递公司信息(kuaidicom)、订单数量(count)、店铺ID(siid)、模板ID(tempId)、寄件人信息(sendManInfo)、收件人信息(recManInfo)、打印类型(printType)等。接着,将orderReq对象序列化成json字符串,并创建一个PrintReq对象,设置PrintReq对象的一些属性,包括请求方法(method)、请求参数(param)、请求时间(t)、签名(sign)等。最后,创建一个LabelV2对象,并调用其execute方法,将PrintReq对象传入,从而完成订单打印。
相关问题
用java代码创建名为学号+姓名首字母的数据表,字段包括id,name,age,其中应有个人信息,使用JDBC知识,根据id更新姓名,更新后的姓名中需有学号,如202220101.张三 20220101java代码
好的,这是一个比较典型的JDBC操作,可以按照以下步骤实现:
1. 导入JDBC相关的jar包,比如mysql-connector-java。
2. 建立数据库连接,这里假设数据库名为test,用户名为root,密码为123456,连接地址为localhost:3306。
```
String url = "jdbc:mysql://localhost:3306/test";
String user = "root";
String password = "123456";
Connection conn = DriverManager.getConnection(url, user, password);
```
3. 创建数据表,需要使用CREATE TABLE语句,这里可以使用String.format方法构建SQL语句。
```
String tableName = "20220101" + name.substring(0, 1);
String createTableSql = String.format(
"CREATE TABLE %s (id INT PRIMARY KEY, name VARCHAR(50), age INT)", tableName);
Statement stmt = conn.createStatement();
stmt.executeUpdate(createTableSql);
```
4. 插入个人信息,使用INSERT INTO语句。
```
String insertSql = String.format(
"INSERT INTO %s (id, name, age) VALUES (%d, '%s', %d)",
tableName, id, name, age);
stmt.executeUpdate(insertSql);
```
5. 更新姓名,使用UPDATE语句。
```
String updateSql = String.format(
"UPDATE %s SET name='%s' WHERE id=%d", tableName, newName, id);
stmt.executeUpdate(updateSql);
```
6. 关闭数据库连接。
```
stmt.close();
conn.close();
```
完整的代码实现如下:
```
import java.sql.*;
public class Main {
public static void main(String[] args) throws Exception {
String url = "jdbc:mysql://localhost:3306/test";
String user = "root";
String password = "123456";
Connection conn = DriverManager.getConnection(url, user, password);
int id = 202220101;
String name = "张三";
int age = 20;
String tableName = "20220101" + name.substring(0, 1);
String createTableSql = String.format(
"CREATE TABLE %s (id INT PRIMARY KEY, name VARCHAR(50), age INT)", tableName);
Statement stmt = conn.createStatement();
stmt.executeUpdate(createTableSql);
String insertSql = String.format(
"INSERT INTO %s (id, name, age) VALUES (%d, '%s', %d)",
tableName, id, name, age);
stmt.executeUpdate(insertSql);
String newName = "20220101" + "李四";
String updateSql = String.format(
"UPDATE %s SET name='%s' WHERE id=%d", tableName, newName, id);
stmt.executeUpdate(updateSql);
stmt.close();
conn.close();
}
}
```
使用e.getMessage()编写下列Java程序: 定义一个"年龄异常类":AgeException,使其继承自Exception,并添加无参、String参数的构造方法; 定义一个"性别异常类":SexException,使其继承自Exception,并添加无参、String参数的构造方法; 定义一个Student类,属性:姓名、性别、年龄。 在性别的set方法中判断是否是男/女,如果不是则抛出:性别异常 在年龄的set方法中判断年龄是否是15–50之间,如果不是则抛出:年龄异常 编写测试类,创建一个Student对象,并在try{}中调用setXxx()方法为对象属性赋值,在catch()中打印年龄错误。 注意:需要使用e.getMessage()
以下是使用e.getMessage()编写的Java程序:
AgeException.java
```
public class AgeException extends Exception {
public AgeException() {}
public AgeException(String message) {
super(message);
}
}
```
SexException.java
```
public class SexException extends Exception {
public SexException() {}
public SexException(String message) {
super(message);
}
}
```
Student.java
```
public class Student {
private String name;
private String sex;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) throws SexException {
if (!sex.equals("男") && !sex.equals("女")) {
throw new SexException("性别异常");
}
this.sex = sex;
}
public int getAge() {
return age;
}
public void setAge(int age) throws AgeException {
if (age < 15 || age > 50) {
throw new AgeException("年龄异常");
}
this.age = age;
}
}
```
Test.java
```
public class Test {
public static void main(String[] args) {
Student s = new Student();
try {
s.setName("张三");
s.setSex("人妖");
s.setAge(60);
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
```
在测试类中,我们创建了一个Student对象,并在try块中调用setXxx()方法为其属性赋值,在catch块中使用e.getMessage()打印异常信息。如果出现年龄异常或性别异常,就会打印对应的错误信息。
阅读全文
相关推荐



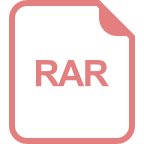












