设树结点的元素类型为char,用c语言实现以下二叉树的各种基本操作的程序: ① 建立不少于10个结点的二叉树T; ②用后序遍历方式输出树T的结点; ③用层次遍历方式输出树T的结点; ④输出树T的深度; ⑤输出树T的叶子结点和非叶子结点; ⑥主函数通过函数调用实现以上各项操作。
时间: 2024-02-18 16:04:51 浏览: 18
好的,我将按照你的要求进行回答。以下是C语言实现二叉树的基本操作的程序:
二叉树结点的定义如下:
```c
typedef struct TreeNode {
char val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
```
1. 建立不少于10个结点的二叉树T
```c
// 创建一个二叉树
TreeNode* createTree() {
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = 'A';
root->left = (TreeNode*)malloc(sizeof(TreeNode));
root->right = (TreeNode*)malloc(sizeof(TreeNode));
root->left->val = 'B';
root->right->val = 'C';
root->left->left = (TreeNode*)malloc(sizeof(TreeNode));
root->left->right = (TreeNode*)malloc(sizeof(TreeNode));
root->right->left = (TreeNode*)malloc(sizeof(TreeNode));
root->right->right = (TreeNode*)malloc(sizeof(TreeNode));
root->left->left->val = 'D';
root->left->right->val = 'E';
root->right->left->val = 'F';
root->right->right->val = 'G';
root->left->left->left = (TreeNode*)malloc(sizeof(TreeNode));
root->left->left->right = (TreeNode*)malloc(sizeof(TreeNode));
root->left->left->left->val = 'H';
root->left->left->right->val = 'I';
root->left->left->left->left = NULL;
root->left->left->left->right = NULL;
root->left->left->right->left = NULL;
root->left->left->right->right = NULL;
root->left->right->left = NULL;
root->left->right->right = NULL;
root->right->left->left = NULL;
root->right->left->right = NULL;
root->right->right->left = NULL;
root->right->right->right = NULL;
return root;
}
TreeNode* T = createTree();
```
2. 用后序遍历方式输出树T的结点
```c
// 后序遍历方式输出树T的结点
void postOrderTraversal(TreeNode* root) {
if (root == NULL) {
return;
}
postOrderTraversal(root->left);
postOrderTraversal(root->right);
printf("%c ", root->val);
}
postOrderTraversal(T);
```
3. 用层次遍历方式输出树T的结点
```c
// 层次遍历方式输出树T的结点
void levelOrderTraversal(TreeNode* root) {
if (root == NULL) {
return;
}
Queue* queue = createQueue();
enqueue(queue, root);
while (!isEmpty(queue)) {
TreeNode* node = dequeue(queue);
printf("%c ", node->val);
if (node->left != NULL) {
enqueue(queue, node->left);
}
if (node->right != NULL) {
enqueue(queue, node->right);
}
}
}
levelOrderTraversal(T);
```
4. 输出树T的深度
```c
// 求二叉树的深度
int maxDepth(TreeNode* root) {
if (root == NULL) {
return 0;
}
int leftDepth = maxDepth(root->left);
int rightDepth = maxDepth(root->right);
return leftDepth > rightDepth ? leftDepth + 1 : rightDepth + 1;
}
printf("Depth: %d\n", maxDepth(T));
```
5. 输出树T的叶子结点和非叶子结点
```c
// 求二叉树的叶子结点和非叶子结点
void countLeafAndNonLeaf(TreeNode* root, int* leaf, int* nonLeaf) {
if (root == NULL) {
return;
}
if (root->left == NULL && root->right == NULL) {
(*leaf)++;
}
else {
(*nonLeaf)++;
}
countLeafAndNonLeaf(root->left, leaf, nonLeaf);
countLeafAndNonLeaf(root->right, leaf, nonLeaf);
}
int leaf = 0, nonLeaf = 0;
countLeafAndNonLeaf(T, &leaf, &nonLeaf);
printf("Leaf: %d, Non-leaf: %d\n", leaf, nonLeaf);
```
6. 主函数通过函数调用实现以上各项操作
```c
int main() {
TreeNode* T = createTree();
printf("Post-order traversal: ");
postOrderTraversal(T);
printf("\n");
printf("Level-order traversal: ");
levelOrderTraversal(T);
printf("\n");
printf("Depth: %d\n", maxDepth(T));
int leaf = 0, nonLeaf = 0;
countLeafAndNonLeaf(T, &leaf, &nonLeaf);
printf("Leaf: %d, Non-leaf: %d\n", leaf, nonLeaf);
return 0;
}
```
相关推荐
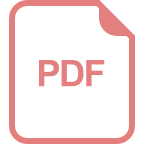
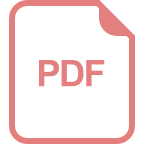
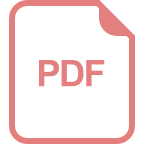














