帮我用python写一个带界面的用摄像头实时识别人脸的代码
时间: 2024-03-16 19:46:52 浏览: 64
好的,您需要安装以下库:
- OpenCV:用于图像处理和人脸识别
- PyQt5:用于创建GUI界面
以下是一份基于 PyQt5 和 OpenCV 的实时人脸识别代码,您可以根据您的需要进行修改:
```python
import cv2
from PyQt5.QtGui import QImage, QPixmap
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel
from PyQt5.QtCore import Qt, QTimer
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("实时人脸识别")
self.setGeometry(100, 100, 640, 480)
# 创建一个标签用于显示摄像头捕捉到的图像
self.image_label = QLabel(self)
self.image_label.setAlignment(Qt.AlignCenter)
self.setCentralWidget(self.image_label)
# 创建一个计时器,每隔一定时间更新一次图像
self.timer = QTimer()
self.timer.timeout.connect(self.update_frame)
self.timer.start(30)
# 创建一个 OpenCV 的人脸识别器
self.face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + "haarcascade_frontalface_default.xml")
# 创建一个摄像头捕捉器
self.capture = cv2.VideoCapture(0)
def update_frame(self):
ret, frame = self.capture.read()
if not ret:
return
# 将 OpenCV 的图像转换为 PyQt5 的图像
rgb_image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
h, w, ch = rgb_image.shape
bytes_per_line = ch * w
qt_image = QImage(rgb_image.data, w, h, bytes_per_line, QImage.Format_RGB888)
# 在图像上进行人脸识别
gray_image = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces = self.face_cascade.detectMultiScale(gray_image, scaleFactor=1.2, minNeighbors=5)
# 在图像上标记人脸
for (x, y, w, h) in faces:
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 0, 255), 2)
# 将带有人脸标记的图像转换为 PyQt5 的图像
rgb_image_with_faces = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
h, w, ch = rgb_image_with_faces.shape
bytes_per_line = ch * w
qt_image_with_faces = QImage(rgb_image_with_faces.data, w, h, bytes_per_line, QImage.Format_RGB888)
# 在 GUI 界面上显示图像
self.image_label.setPixmap(QPixmap.fromImage(qt_image_with_faces))
if __name__ == "__main__":
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
运行代码后,将会打开一个 GUI 窗口,其中包含一个摄像头捕捉到的图像和一个人脸识别后带有标记的图像。
阅读全文
相关推荐
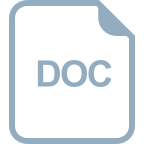
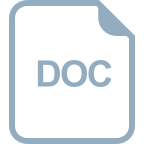
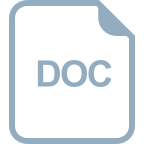
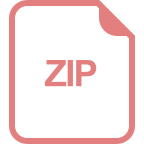
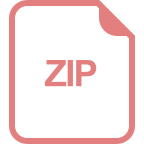
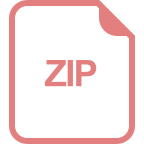



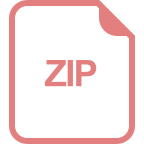
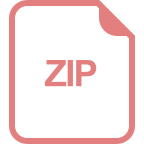
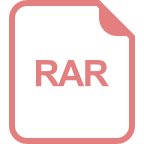






