我想通过free -m 获取当前系统的内存使用率c++代码实现
时间: 2024-12-11 18:44:37 浏览: 18
要通过C++代码获取系统内存使用率,通常需要利用操作系统提供的接口或者第三方库。然而,直接在C++中执行shell命令`free -m`是不现实的,因为它涉及到外部命令执行。你可以选择以下两种方法:
**1. 使用`psutil`库**
如果你的应用支持安装额外的库,可以考虑使用`psutil`库,这是一个跨平台的Python模块,但它也提供了C++ API。首先,你需要将`psutil`引入到C++项目中,然后编写代码如下:
```cpp
#include <iostream>
#include "psutil.hpp"
int main() {
try {
std::vector<std::string> memory_usage_lines = psutil::virtual_memory().as_vector();
for (const auto& line : memory_usage_lines) {
if (line.find("% used") != std::string::npos) {
double percent_used = std::stod(line.substr(line.find("% used") + 6));
std::cout << "Memory Usage: " << percent_used << "%\n";
break; // Assuming you only need the first line with % used information
}
}
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << '\n';
}
return 0;
}
```
确保先安装了`libpsutil-dev`(如果是Linux)或对应版本的Windows库。
**2. 利用C++17的Process API(仅限Unix-like系统)**
从C++17开始,标准库提供了一些功能,可以用C++直接访问系统信息。但在Linux等Unix-like系统中,可以尝试使用`std::filesystem::executors`来运行shell命令并捕获输出:
```cpp
#include <iostream>
#include <filesystem>
std::optional<int> get_memory_usage() {
try {
std::string output;
std::error_code ec;
auto result = std::filesystem::execute(
{"/bin/bash", "-c", "free -m | awk 'NR==2{print $3/$2 * 100}'"},
std::redirect_stdout,
std::redirect_error,
ec);
if (!ec) {
std::istringstream iss(output);
int mem_usage;
iss >> mem_usage;
return mem_usage;
}
else {
throw std::runtime_error("Failed to execute free command: " + ec.message());
}
}
catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << '\n';
return std::nullopt;
}
}
int main() {
auto mem_usage = get_memory_usage();
if (mem_usage.has_value()) {
std::cout << "Memory Usage: " << mem_usage.value() << "%\n";
}
return 0;
}
```
请注意,这些方法都依赖于特定的操作系统环境,并非跨平台适用。如果需要跨平台,还是推荐使用像`psutil`这样的库。
阅读全文
相关推荐



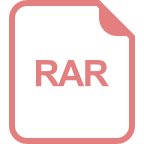
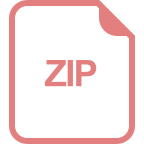

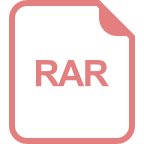

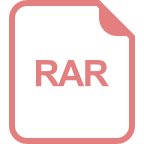









