获取amd的GPU使用率,使用C++代码
时间: 2024-05-01 13:18:11 浏览: 18
你可以使用 AMD GPU Profiler 工具来获取 AMD GPU 的使用率。以下是获取 AMD GPU 使用率的示例 C 代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// AMD GPU Profiler的头文件
#include "CL/CL.h"
#include "CPerfCounter.h"
int main()
{
int deviceCount = 0;
cl_device_id* devices = NULL;
cl_ulong maxClockFrequency = 0;
cl_uint numOfComputeUnits = 0;
cl_platform_id platformId = 0;
clGetPlatformIDs(1, &platformId, NULL);
// 获取所有的AMD GPU设备
clGetDeviceIDs(platformId, CL_DEVICE_TYPE_GPU, 0, NULL, &deviceCount);
devices = (cl_device_id*)malloc(sizeof(cl_device_id) * deviceCount);
clGetDeviceIDs(platformId, CL_DEVICE_TYPE_GPU, deviceCount, devices, NULL);
for (int i = 0; i < deviceCount; ++i)
{
cl_device_id device = devices[i];
// 获取设备的运行包络信息
cl_ulong maxClockFrequency = 0;
clGetDeviceInfo(device, CL_DEVICE_MAX_CLOCK_FREQUENCY, sizeof(cl_ulong), &maxClockFrequency, NULL);
cl_uint numOfComputeUnits = 0;
clGetDeviceInfo(device, CL_DEVICE_MAX_COMPUTE_UNITS, sizeof(cl_uint), &numOfComputeUnits, NULL);
// 创建 OpenCL 上下文
cl_context_properties cps[3] = { CL_CONTEXT_PLATFORM, (cl_context_properties)platformId, 0 };
cl_context context = clCreateContext(cps, 1, &device, NULL, NULL, NULL);
// 创建命令队列
cl_command_queue commandQueue = clCreateCommandQueue(context, device, CL_QUEUE_PROFILING_ENABLE, NULL);
// 创建 Profiling 对象
CPerfCounter timer(numOfComputeUnits, commandQueue);
// 创建程序对象和内核对象
const char* kernelSource = "__kernel void gpuUtil(__global float* result)\n"
"{\n"
" int tid = get_global_id(0);\n"
" float a = 0.0f;\n"
" while (true)\n"
" {\n"
" a += tid * 0.1f;\n"
" result[tid] = a;\n"
" }\n"
"}\n";
cl_program program = clCreateProgramWithSource(context, 1, &kernelSource, NULL, NULL);
clBuildProgram(program, 1, &device, NULL, NULL, NULL);
cl_kernel kernel = clCreateKernel(program, "gpuUtil", NULL);
// 创建输出数据缓冲区
cl_mem output = clCreateBuffer(context, CL_MEM_WRITE_ONLY | CL_MEM_ALLOC_HOST_PTR, sizeof(float) * numOfComputeUnits, NULL, NULL);
// 设置内核参数
clSetKernelArg(kernel, 0, sizeof(cl_mem), &output);
// 启动内核
size_t global_work_size[] = { numOfComputeUnits };
clEnqueueNDRangeKernel(commandQueue, kernel, 1, NULL, global_work_size, NULL, 0, NULL, NULL);
// 读取结果
float* result = (float*)clEnqueueMapBuffer(commandQueue, output, CL_TRUE, CL_MAP_READ, 0, sizeof(float) * numOfComputeUnits, 0, NULL, NULL, NULL);
// 计算 GPU 使用率
double gpuUtilization = timer.GetUtilization(result);
printf("AMD GPU %d - Utilization: %.2f%%\n", i + 1, gpuUtilization);
// 清理资源
clEnqueueUnmapMemObject(commandQueue, output, result, 0, NULL, NULL);
clReleaseMemObject(output);
clReleaseKernel(kernel);
clReleaseProgram(program);
clReleaseCommandQueue(commandQueue);
clReleaseContext(context);
}
free(devices);
return 0;
}
```
在上面的示例代码中,我们通过 AMD GPU Profiler 工具来获取GPU使用率。它利用了AMD GPU Profiler的函数库和CPerfCounter类库来计算GPU使用率,并利用OpenCL技术进行核操作。需要注意的是,该示例代码只是一个简单的演示,仅供参考,需要针对具体的GPU进行优化和调整。
相关推荐
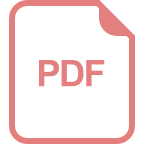
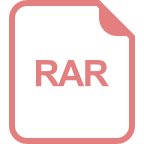
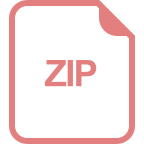














