vue 根据当前位置获取天气信息
时间: 2023-09-30 09:10:15 浏览: 80
要根据当前位置获取天气信息,你需要使用浏览器的定位功能来获取用户的经纬度,然后将经纬度传递给一个天气 API 接口,获取天气信息。
以下是一个使用 Vue.js 实现这一功能的示例:
首先,你需要使用 HTML5 的 Geolocation API 来获取用户的当前位置信息,代码如下:
```javascript
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(position => {
const { latitude, longitude } = position.coords
// 在这里调用天气 API 接口,传递经纬度参数
})
} else {
console.error("Geolocation is not supported by this browser.")
}
```
接下来,你需要调用一个天气 API 接口来获取天气信息。这里我们使用 OpenWeatherMap 提供的免费天气 API 接口。你可以在 OpenWeatherMap 注册一个账号,然后获取一个 API Key。
以下是一个可以获取天气信息的 Vue.js 组件,代码中使用了 axios 库来发送 HTTP 请求:
```vue
<template>
<div>
<h1>{{ city }}</h1>
<p>{{ description }}</p>
<p>{{ temperature }}°C</p>
</div>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
city: '',
description: '',
temperature: ''
}
},
mounted() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(position => {
const { latitude, longitude } = position.coords
const apiKey = 'your-api-key' // 替换成你自己的 OpenWeatherMap API Key
const apiUrl = `https://api.openweathermap.org/data/2.5/weather?lat=${latitude}&lon=${longitude}&appid=${apiKey}&units=metric`
axios.get(apiUrl)
.then(response => {
const { name, weather, main } = response.data
this.city = name
this.description = weather[0].description
this.temperature = main.temp
})
.catch(error => {
console.error(error)
})
})
} else {
console.error("Geolocation is not supported by this browser.")
}
}
}
</script>
```
在上面的代码中,我们使用了 Vue.js 的 data 属性来存储获取到的天气信息,并在模板中展示出来。在组件的 mounted 钩子函数中,我们调用了浏览器的 Geolocation API 获取用户的位置信息,并将经纬度参数传递给 OpenWeatherMap 的 API 接口,获取天气信息。在获取成功后,我们更新 data 属性中的值,以便在模板中展示出来。
你可以将上面的代码复制到一个 Vue.js 单文件组件中,然后在你的应用程序中使用这个组件,就可以根据用户的位置获取天气信息了。
相关推荐
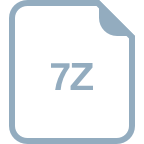














