C语言实现SJF作业调度算法
时间: 2024-05-04 19:01:39 浏览: 73
Sure, SJF(Shortest Job First) algorithm is a scheduling algorithm, in which the process having the smallest execution time is chosen for the next execution. Here's a sample code for implementing SJF scheduling algorithm in C language:
```
#include <stdio.h>
#include <stdlib.h>
int main() {
int n, i, j, pos, temp;
float avg_wt, avg_tt, total_wt = 0, total_tt = 0;
printf("Enter number of processes: ");
scanf("%d", &n);
int bt[n], wt[n], tt[n];
printf("Enter burst time for each process:\n");
for(i=0;i<n;i++) {
printf("Process %d: ", (i+1));
scanf("%d", &bt[i]);
}
// sorting the burst time in ascending order
for(i=0;i<n;i++) {
pos = i;
for(j=i+1;j<n;j++) {
if(bt[j] < bt[pos]) {
pos = j;
}
}
temp = bt[i];
bt[i] = bt[pos];
bt[pos] = temp;
}
wt[0] = 0;
// calculating waiting time for each process
for(i=1;i<n;i++) {
wt[i] = 0;
for(j=0;j<i;j++) {
wt[i] += bt[j];
}
total_wt += wt[i];
}
avg_wt = (float)total_wt / n;
// calculating turnaround time for each process
for(i=0;i<n;i++) {
tt[i] = bt[i] + wt[i];
total_tt += tt[i];
}
avg_tt = (float)total_tt / n;
// output
printf("\nSJF Scheduling Algorithm");
printf("\n------------------------");
printf("\nProcess\tBurst Time\tWaiting Time\tTurnaround Time\n");
for(i=0;i<n;i++) {
printf("%d\t\t%d\t\t%d\t\t%d\n", (i+1), bt[i], wt[i], tt[i]);
}
printf("\nAverage waiting time: %.2f", avg_wt);
printf("\nAverage turnaround time: %.2f", avg_tt);
return 0;
}
```
Hope this helps!
阅读全文
相关推荐
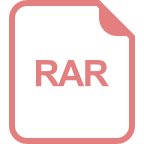
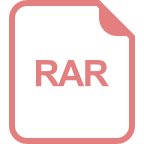
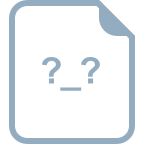















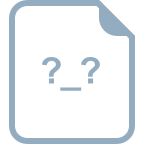