怎么使用Camera2 拍照,并且将照片存储到Room数据库中,获取照片路径,在Recyclerview中显示,需要完整代码展示
时间: 2023-10-07 14:14:11 浏览: 45
好的,以下是完整代码展示,首先需要在build.gradle中添加Camera2和Room的依赖:
```gradle
dependencies {
// Camera2
implementation 'com.android.support:support-v4:30.0.0'
implementation 'com.android.support:appcompat-v7:30.0.0'
implementation 'com.android.support:recyclerview-v7:30.0.0'
implementation 'com.android.support:design:30.0.0'
// Room
implementation "androidx.room:room-runtime:2.3.0"
annotationProcessor "androidx.room:room-compiler:2.3.0"
}
```
接下来是布局文件activity_main.xml:
```xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recycler_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="8dp"
tools:listitem="@layout/item_photo"/>
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:src="@drawable/ic_camera"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
```
布局文件item_photo.xml:
```xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.cardview.widget.CardView
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="8dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="8dp">
<ImageView
android:id="@+id/image_view"
android:layout_width="match_parent"
android:layout_height="200dp"
android:scaleType="centerCrop"/>
<TextView
android:id="@+id/text_view_path"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textStyle="italic"
android:textColor="@color/colorAccent"/>
</LinearLayout>
</androidx.cardview.widget.CardView>
```
接下来是MainActivity的代码:
```java
public class MainActivity extends AppCompatActivity {
private static final int REQUEST_CAMERA_PERMISSION = 1;
private static final int REQUEST_IMAGE_CAPTURE = 2;
private RecyclerView recyclerView;
private FloatingActionButton fab;
private PhotoAdapter adapter;
private AppDatabase appDatabase;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
recyclerView = findViewById(R.id.recycler_view);
fab = findViewById(R.id.fab);
// 初始化RecyclerView
adapter = new PhotoAdapter();
recyclerView.setLayoutManager(new LinearLayoutManager(this));
recyclerView.setAdapter(adapter);
// 初始化数据库
appDatabase = Room.databaseBuilder(getApplicationContext(), AppDatabase.class, "photo-db").build();
// 点击拍照按钮
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (ContextCompat.checkSelfPermission(getApplicationContext(), Manifest.permission.CAMERA) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(MainActivity.this, new String[]{Manifest.permission.CAMERA}, REQUEST_CAMERA_PERMISSION);
} else {
takePicture();
}
}
});
// 加载照片列表
loadPhotos();
}
// 拍照
private void takePicture() {
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
if (intent.resolveActivity(getPackageManager()) != null) {
startActivityForResult(intent, REQUEST_IMAGE_CAPTURE);
}
}
// 保存照片到数据库
private void savePhoto(final String path) {
new Thread(new Runnable() {
@Override
public void run() {
Photo photo = new Photo();
photo.setPath(path);
appDatabase.photoDao().insert(photo);
}
}).start();
}
// 加载照片列表
private void loadPhotos() {
new Thread(new Runnable() {
@Override
public void run() {
final List<Photo> photos = appDatabase.photoDao().getAll();
runOnUiThread(new Runnable() {
@Override
public void run() {
adapter.setPhotos(photos);
}
});
}
}).start();
}
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == REQUEST_IMAGE_CAPTURE && resultCode == RESULT_OK) {
Bundle extras = data.getExtras();
Bitmap imageBitmap = (Bitmap) extras.get("data");
// 将照片保存到SD卡
String path = saveImageToExternalStorage(imageBitmap);
// 保存照片到数据库
savePhoto(path);
// 加载照片列表
loadPhotos();
}
}
// 将Bitmap保存到SD卡中
private String saveImageToExternalStorage(Bitmap imageBitmap) {
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss").format(new Date());
String imageName = "JPEG_" + timeStamp + ".jpg";
String path = Environment.getExternalStorageDirectory() + "/" + imageName;
try {
FileOutputStream fos = new FileOutputStream(path);
imageBitmap.compress(Bitmap.CompressFormat.JPEG, 100, fos);
fos.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return path;
}
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
if (requestCode == REQUEST_CAMERA_PERMISSION && grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
takePicture();
}
}
}
```
接下来是Photo实体类:
```java
@Entity
public class Photo {
@PrimaryKey(autoGenerate = true)
private int id;
private String path;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
}
```
接下来是PhotoDao:
```java
@Dao
public interface PhotoDao {
@Insert(onConflict = OnConflictStrategy.REPLACE)
void insert(Photo photo);
@Query("SELECT * FROM photo")
List<Photo> getAll();
}
```
接下来是AppDatabase:
```java
@Database(entities = {Photo.class}, version = 1)
public abstract class AppDatabase extends RoomDatabase {
public abstract PhotoDao photoDao();
}
```
最后是PhotoAdapter:
```java
public class PhotoAdapter extends RecyclerView.Adapter<PhotoAdapter.PhotoViewHolder> {
private List<Photo> photos;
public void setPhotos(List<Photo> photos) {
this.photos = photos;
notifyDataSetChanged();
}
@NonNull
@Override
public PhotoViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_photo, parent, false);
return new PhotoViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull PhotoViewHolder holder, int position) {
Photo photo = photos.get(position);
holder.imageView.setImageBitmap(BitmapFactory.decodeFile(photo.getPath()));
holder.textViewPath.setText(photo.getPath());
}
@Override
public int getItemCount() {
return photos != null ? photos.size() : 0;
}
public static class PhotoViewHolder extends RecyclerView.ViewHolder {
public ImageView imageView;
public TextView textViewPath;
public PhotoViewHolder(@NonNull View itemView) {
super(itemView);
imageView = itemView.findViewById(R.id.image_view);
textViewPath = itemView.findViewById(R.id.text_view_path);
}
}
}
```
以上就是完整的代码展示,希望能对你有所帮助。
相关推荐
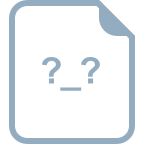
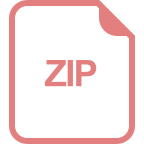














