c 语言 设计一个子函数,统计任意一串字符中数字字符的个数,并在主函数中调用此函数。
时间: 2024-05-01 19:22:52 浏览: 81
可以使用如下的代码来实现:
```c
#include <stdio.h>
int count_digits(char* str) {
int count = 0;
while (*str) {
if (*str >= '0' && *str <= '9') {
count++;
}
str++;
}
return count;
}
int main() {
char str[100];
printf("请输入一个字符串:");
scanf("%s", str);
int count = count_digits(str);
printf("数字字符的个数为:%d\n", count);
return 0;
}
```
首先在主函数中输入一个字符串,然后调用 `count_digits` 函数来统计其中数字字符的个数。在 `count_digits` 函数中,我们使用一个 while 循环来遍历字符串中的每一个字符,如果字符是数字字符,就将计数器加一。最后返回计数器的值即可。
相关问题
c语言 设计一个子函数,统计任意一串字符中数字字符的个数,并在主函数中调用此函数
实现如下:
```c
#include <stdio.h>
int count_digits(char *str) {
int count = 0;
while (*str != '\0') {
if (*str >= '0' && *str <= '9') {
count++;
}
str++;
}
return count;
}
int main() {
char str[100];
printf("请输入任意一串字符:");
fgets(str, 100, stdin);
int count = count_digits(str);
printf("数字字符的个数为:%d\n", count);
return 0;
}
```
这段代码中,`count_digits` 函数用来统计字符串中数字字符的个数。在主函数中,我们先输入一串字符,然后调用 `count_digits` 函数进行统计,并输出结果。
c语言设计一个子函数,统计任意一串字符中数字字符的个数,并在主函数中调用此函数。
下面是一个简单的示例代码:
```c
#include <stdio.h>
int count_digits(char str[]) {
int count = 0;
for (int i = 0; str[i] != '\0'; i++) {
if (str[i] >= '0' && str[i] <= '9') {
count++;
}
}
return count;
}
int main() {
char str[100];
printf("请输入一串字符:");
scanf("%s", str);
int count = count_digits(str);
printf("数字字符的个数为:%d\n", count);
return 0;
}
```
在上面的代码中,我们首先定义了一个名为 `count_digits` 的函数,该函数接收一个字符串作为参数,并返回其中数字字符的个数。在函数内部,我们使用了一个 `for` 循环来遍历字符串中的每个字符,如果当前字符是数字字符,则将计数器 `count` 加一。最后,函数返回计数器的值。
在主函数中,我们首先定义了一个字符数组 `str`,并使用 `scanf` 函数从标准输入中读取用户输入的字符串。接着,我们调用了 `count_digits` 函数来统计字符串中数字字符的个数,并将结果保存在变量 `count` 中。最后,我们输出了计数器的值。
阅读全文
相关推荐
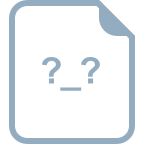










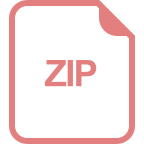