不相等的存入list,相等的存入子list
时间: 2024-01-15 08:43:10 浏览: 66
可以使用一个字典来实现这个功能,遍历列表,如果元素不在字典的键中,就将其作为键加入字典,并将其值初始化为一个列表,将该元素存入该列表。如果元素已经在字典的键中,就将其添加到对应的列表中。最后,将字典中所有值为单个元素的列表转换为非嵌套列表即可。
代码示例:
```
def split_list(lst):
d = {}
for item in lst:
if item not in d:
d[item] = [item]
else:
d[item].append(item)
return [v if len(v) > 1 else v[0] for v in d.values()]
# 测试
lst = [1, 2, 3, 2, 4, 5, 4, 6, 7, 7]
result = split_list(lst)
print(result)
# 输出:[[2, 2], [4, 4], [7, 7], 1, 3, 5, 6]
```
相关问题
假如我已经得出两个表的List对象,用java如何关联两个表取出取出id相等的对象并存入List中
假设你有两个表的List对象,每个表中都有一个id属性,你可以使用Java 8的Stream API来实现关联两个表并取出id相等的对象,代码示例如下:
```java
List<Table1> table1List = ...; // 第一个表的List对象
List<Table2> table2List = ...; // 第二个表的List对象
List<Result> resultList = table1List.stream()
.flatMap(table1 -> table2List.stream()
.filter(table2 -> table1.getId() == table2.getId())
.map(table2 -> new Result(table1, table2)))
.collect(Collectors.toList());
```
其中,Result是一个自定义类,用于存储关联后的对象。上述代码中,首先对第一个表的List对象进行遍历,然后对第二个表的List对象进行过滤,只取出id相等的对象,并将两个对象封装成一个Result对象,最后将所有的Result对象收集到一个List中返回。
用滑动窗口的方法遍历一个列表,如果发现列表中有连续3个值相等,则这个值存入一个新的列表
在编程中,可以使用滑动窗口(Sliding Window)算法遍历列表并检查是否有连续三个相同的值。以下是一个简单的Python示例,它创建了一个新列表只包含原列表中连续三个相等的元素:
```python
def find_consecutive_triples(lst):
result = []
window = [lst[0], lst[1], lst[2]] if len(lst) >= 3 else None
# 滑动窗口从第三个元素开始
for i in range(3, len(lst)):
if window == [lst[i - 2], lst[i - 1], lst[i]]:
result.append(window)
else:
window = [lst[i], lst[i + 1], lst[i + 2]]
return result
# 示例
input_list = [1, 2, 2, 3, 4, 5, 6, 7, 8, 8, 8]
output_list = find_consecutive_triples(input_list)
print(output_list)
阅读全文
相关推荐
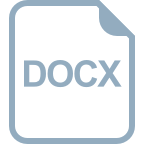
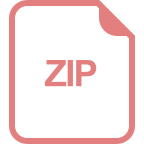
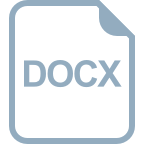












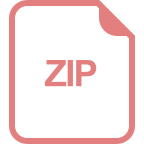
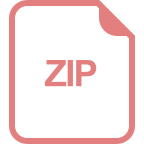