利用android studio来写天气预报代码
时间: 2023-10-06 12:06:34 浏览: 150
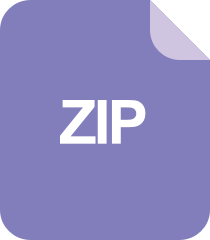
Android Studio项目《天气预报app》

好的,以下是一个简单的天气预报应用程序的代码示例:
首先,在 Android Studio 中创建一个新的项目,并添加以下依赖项:
```
implementation 'com.android.volley:volley:1.2.0'
implementation 'com.squareup.picasso:picasso:2.71828'
```
接下来,在 `AndroidManifest.xml` 文件中添加以下权限:
```xml
<uses-permission android:name="android.permission.INTERNET" />
```
然后,在 `activity_main.xml` 文件中添加以下布局:
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<LinearLayout
android:id="@+id/weather_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:gravity="center_horizontal">
<ImageView
android:id="@+id/weather_icon"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_launcher"/>
<TextView
android:id="@+id/weather_temp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="0 ℃"
android:textSize="48sp"
android:textStyle="bold"/>
<TextView
android:id="@+id/weather_desc"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="晴"/>
</LinearLayout>
</LinearLayout>
```
在 `MainActivity.java` 文件中,添加以下代码:
```java
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.widget.ImageView;
import android.widget.TextView;
import com.android.volley.Request;
import com.android.volley.RequestQueue;
import com.android.volley.Response;
import com.android.volley.VolleyError;
import com.android.volley.toolbox.JsonObjectRequest;
import com.android.volley.toolbox.Volley;
import com.squareup.picasso.Picasso;
import org.json.JSONException;
import org.json.JSONObject;
public class MainActivity extends AppCompatActivity {
private static final String TAG = "MainActivity";
private static final String API_KEY = "YOUR_API_KEY";
private static final String WEATHER_API_URL = "https://api.openweathermap.org/data/2.5/weather?q=Shanghai&units=metric&appid=" + API_KEY;
private ImageView mWeatherIcon;
private TextView mWeatherTemp;
private TextView mWeatherDesc;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mWeatherIcon = findViewById(R.id.weather_icon);
mWeatherTemp = findViewById(R.id.weather_temp);
mWeatherDesc = findViewById(R.id.weather_desc);
RequestQueue requestQueue = Volley.newRequestQueue(this);
JsonObjectRequest jsonObjectRequest = new JsonObjectRequest(Request.Method.GET, WEATHER_API_URL, null, new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
try {
JSONObject main = response.getJSONObject("main");
String temp = main.getString("temp") + " ℃";
mWeatherTemp.setText(temp);
String icon = response.getJSONArray("weather").getJSONObject(0).getString("icon");
String iconUrl = "https://openweathermap.org/img/w/" + icon + ".png";
Picasso.get().load(iconUrl).into(mWeatherIcon);
String desc = response.getJSONArray("weather").getJSONObject(0).getString("main");
mWeatherDesc.setText(desc);
} catch (JSONException e) {
e.printStackTrace();
}
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
Log.e(TAG, "onErrorResponse: ", error);
}
});
requestQueue.add(jsonObjectRequest);
}
}
```
在代码中,我们使用了 `Volley` 库来进行网络请求,获取天气数据。同时,我们还使用了 `Picasso` 库来加载天气图标。
请注意替换 `API_KEY` 为你自己的 OpenWeatherMap API Key,并将 `WEATHER_API_URL` 中的城市名称更改为你想要查询的城市。
完成后,运行应用程序,即可看到简单的天气预报应用程序。
阅读全文
相关推荐
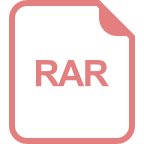
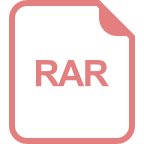











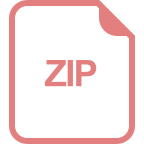
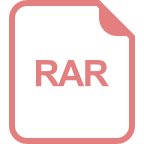
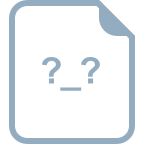
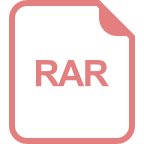
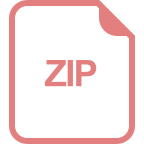