js 格式化时间为字符串
时间: 2023-12-23 21:02:46 浏览: 24
可以使用 JavaScript 的内置函数 `toLocaleString()` 来格式化时间为字符串。以下是一个例子,将当前时间格式化为 yyyy-mm-dd hh:mm:ss 的字符串:
```javascript
const now = new Date();
const formattedTime = now.toLocaleString("zh-CN", {
year: "numeric",
month: "2-digit",
day: "2-digit",
hour: "2-digit",
minute: "2-digit",
second: "2-digit",
});
console.log(formattedTime); // 输出类似 "2022-01-01 12:34:56" 的字符串
```
其中,第一个参数 `"zh-CN"` 是指定语言和国家,可以根据需要修改。第二个参数是一个对象,用于指定时间的格式。可以根据需要添加或删除属性,比如如果不需要秒数,可以删除 `second: "2-digit"`。
相关问题
js 日期字符串 格式化
在 JavaScript 中,可以使用以下方法来对日期字符串进行格式化。
首先,我们需要将日期字符串转换为 JavaScript 的 Date 对象。
```javascript
var dateString = "2022-10-05";
var date = new Date(dateString);
```
接下来,我们可以使用 `Date` 对象的相关方法来获取日期的各个部分。
```javascript
var year = date.getFullYear();
var month = date.getMonth() + 1; // 月份从 0 开始,所以需要加 1
var day = date.getDate();
var hours = date.getHours();
var minutes = date.getMinutes();
var seconds = date.getSeconds();
```
然后,我们可以根据需要来组织日期字符串的格式。以下是一些常见的日期格式化方式。
1. 年-月-日
```javascript
var formattedDateString = year + "-" + month + "-" + day;
```
2. 月/日/年
```javascript
var formattedDateString = month + "/" + day + "/" + year;
```
3. 日期和时间
```javascript
var formattedDateString = year + "-" + month + "-" + day + " " + hours + ":" + minutes + ":" + seconds;
```
还可以使用内置的 `toLocaleString()` 方法来自动根据所在地区的语言和时间格式进行日期格式化。
```javascript
var formattedDateString = date.toLocaleString();
```
以上是对日期字符串进行简单格式化的方法。如果需要更复杂的格式化,可以使用第三方库如 `moment.js`。
js字符串转日期格式化
在JavaScript中,可以使用Date对象和一些方法来将字符串转换为日期格式。
第一种方法是使用`new Date()`构造函数。你可以直接将字符串传递给构造函数,它会根据字符串的格式来生成相应的日期对象。例如,如果你有一个表示日期的字符串"2022-07-28",你可以使用以下代码将其转换为日期对象:
```javascript
let dateString = "2022-07-28";
let dateObj = new Date(dateString);
console.log(dateObj); // 输出:Thu Jul 28 2022 00:00:00 GMT+0800 (中国标准时间)
```
第二种方法是使用日期格式化库,如moment.js。这个库提供了更多的日期格式化选项,让你可以根据自己的需求来格式化日期。以下是一个使用moment.js将日期字符串格式化的示例:
```javascript
let dateString = "2022-07-28";
let formattedDate = moment(dateString).format("YYYY-MM-DD");
console.log(formattedDate); // 输出:2022-07-28
```
无论你使用哪种方法,都可以根据需要来格式化日期字符串。注意,在使用moment.js之前,你需要先在项目中引入moment.js库。<span class="em">1</span>
#### 引用[.reference_title]
- *1* [js日期格式化的两种方法](https://blog.csdn.net/qq_45904018/article/details/126031642)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
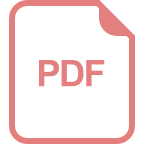
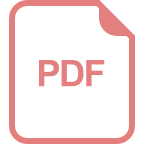








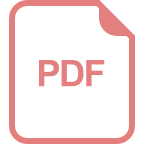
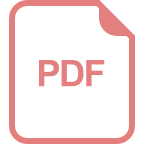
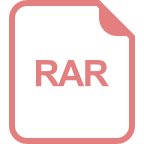