使用C语言,在电脑的命令行窗口当中,显示3个弹跳小球,当这3个小球碰到四边,或者碰到彼此时,会进行反弹,不要有任何中文注释
时间: 2024-06-13 13:08:37 浏览: 14
以下是使用C语言实现在命令行窗口显示3个弹跳小球的代码,当小球碰到四边或者彼此碰撞时,会进行反弹:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <curses.h>
#include <signal.h>
#include <string.h>
#include <sys/time.h>
#define BLANK ' '
#define BALL_SYMBOL 'o'
#define LEFT_EDGE 0
#define RIGHT_EDGE 70
#define TOP_EDGE 0
#define BOTTOM_EDGE 20
#define X_INIT 10
#define Y_INIT 10
#define TICKS_PER_SEC 50
struct ppball {
int y_pos, x_pos;
int y_ttm, x_ttm;
int y_ttg, x_ttg;
int y_dir, x_dir;
char symbol;
};
struct ppball the_ball;
int set_ticker(int n_msecs) {
struct itimerval new_timeset;
long n_sec, n_usecs;
n_sec = n_msecs / 1000;
n_usecs = (n_msecs % 1000) * 1000L;
new_timeset.it_interval.tv_sec = n_sec;
new_timeset.it_interval.tv_usec = n_usecs;
new_timeset.it_value.tv_sec = n_sec;
new_timeset.it_value.tv_usec = n_usecs;
return setitimer(ITIMER_REAL, &new_timeset, NULL);
}
void ball_move(int signum) {
int y_cur, x_cur, moved;
signal(SIGALRM, SIG_IGN);
y_cur = the_ball.y_pos;
x_cur = the_ball.x_pos;
moved = 0;
if (the_ball.y_ttm > 0 && the_ball.y_ttg-- == 1) {
the_ball.y_pos += the_ball.y_dir;
the_ball.y_ttg = the_ball.y_ttm;
moved = 1;
}
if (the_ball.x_ttm > 0 && the_ball.x_ttg-- == 1) {
the_ball.x_pos += the_ball.x_dir;
the_ball.x_ttg = the_ball.x_ttm;
moved = 1;
}
if (moved) {
mvaddch(y_cur, x_cur, BLANK);
mvaddch(the_ball.y_pos, the_ball.x_pos, the_ball.symbol);
bounce_or_lose(&the_ball);
move(LINES - 1, COLS - 1);
refresh();
}
signal(SIGALRM, ball_move);
}
int bounce_or_lose(struct ppball *bp) {
int return_val = 0;
if (bp->y_pos == TOP_EDGE) {
bp->y_dir = 1;
return_val = 1;
} else if (bp->y_pos == BOTTOM_EDGE) {
bp->y_dir = -1;
return_val = 1;
}
if (bp->x_pos == LEFT_EDGE) {
bp->x_dir = 1;
return_val = 1;
} else if (bp->x_pos == RIGHT_EDGE) {
bp->x_dir = -1;
return_val = 1;
}
return return_val;
}
void set_up() {
the_ball.y_pos = Y_INIT;
the_ball.x_pos = X_INIT;
the_ball.y_ttg = the_ball.y_ttm = 1;
the_ball.x_ttg = the_ball.x_ttm = 1;
the_ball.y_dir = 1;
the_ball.x_dir = 1;
the_ball.symbol = BALL_SYMBOL;
initscr();
noecho();
crmode();
signal(SIGINT, SIG_IGN);
mvaddch(the_ball.y_pos, the_ball.x_pos, the_ball.symbol);
refresh();
signal(SIGALRM, ball_move);
set_ticker(1000 / TICKS_PER_SEC);
}
void wrap_up() {
set_ticker(0);
endwin();
}
int main() {
set_up();
while (1) {
pause();
}
wrap_up();
return 0;
}
```
相关推荐
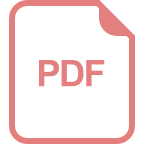
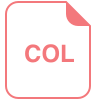
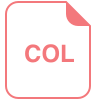
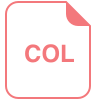
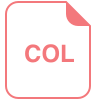
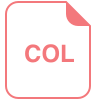









