遗传算法函数优化python
时间: 2023-10-28 21:59:06 浏览: 46
遗传算法是一种基于自然选择和遗传机制的优化算法,可以用来解决函数优化问题。在Python中,你可以使用遗传算法库,如DEAP(Distributed Evolutionary Algorithms in Python)来实现。
以下是一个简单的示例代码,演示如何使用遗传算法来优化一个函数:
```python
import random
from deap import base, creator, tools
# 定义目标函数
def my_function(x):
return x ** 2 + 2 * x + 1
# 定义适应度评价函数
def evaluate(individual):
x = individual[0]
return my_function(x),
# 创建遗传算法工具箱
toolbox = base.Toolbox()
# 创建一个最小化的适应度评价器
creator.create("FitnessMin", base.Fitness, weights=(-1.0,))
# 创建一个个体类,继承于列表
creator.create("Individual", list, fitness=creator.FitnessMin)
# 注册随机浮点数生成器
toolbox.register("attr_float", random.uniform, -10, 10)
# 注册个体生成器
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_float, n=1)
# 注册种群生成器
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
# 注册评价函数
toolbox.register("evaluate", evaluate)
# 注册交叉函数
toolbox.register("mate", tools.cxOnePoint)
# 注册变异函数
toolbox.register("mutate", tools.mutGaussian, mu=0, sigma=1, indpb=0.1)
# 注册选择函数
toolbox.register("select", tools.selTournament, tournsize=3)
def main():
# 设置随机种子
random.seed(0)
# 创建种群
population = toolbox.population(n=50)
# 迭代次数
num_generations = 100
# 评价所有个体
fitnesses = map(toolbox.evaluate, population)
for ind, fit in zip(population, fitnesses):
ind.fitness.values = fit
# 进化的主循环
for g in range(num_generations):
# 选择下一代个体
offspring = toolbox.select(population, len(population))
# 克隆选中个体
offspring = list(map(toolbox.clone, offspring))
# 对选中个体进行交叉和变异操作
for child1, child2 in zip(offspring[::2], offspring[1::2]):
toolbox.mate(child1, child2)
toolbox.mutate(child1)
toolbox.mutate(child2)
del child1.fitness.values
del child2.fitness.values
# 评价新生成的个体
invalid_ind = [ind for ind in offspring if not ind.fitness.valid]
fitnesses = map(toolbox.evaluate, invalid_ind)
for ind, fit in zip(invalid_ind, fitnesses):
ind.fitness.values = fit
# 替换种群中最差的个体
population[:] = toolbox.select(population + offspring, len(population))
# 输出最优解
best_individual = tools.selBest(population, k=1)[0]
best_fitness = best_individual.fitness.values[0]
best_x = best_individual[0]
print("最优解: x =", best_x)
print("最优适应度:", best_fitness)
if __name__ == "__main__":
main()
```
在上面的示例代码中,我们首先定义了一个目标函数`my_function`,然后定义了适应度评价函数`evaluate`,它计算个体的适应度值。接下来,我们使用DEAP创建了遗传算法工具箱,并注册了必要的函数和参数。然后,我们创建了一个种群,并对种群中的个体进行评价。接下来,通过选择、交叉和变异操作,进化出新一代的个体。最后,输出找到的最优解和最优适应度。
这只是一个简单的示例,你可以根据你的具体问题和需求进行相应的修改和扩展。希望对你有帮助!
相关推荐
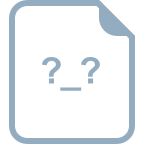
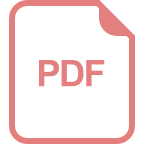
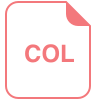
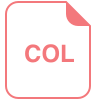
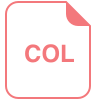
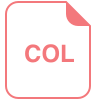
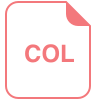









