遗传算法求函数最小值Python
时间: 2023-11-05 09:22:25 浏览: 121
遗传算法是一种基于生物进化原理的优化算法,可以用来求解函数的最小值。下面是一个使用遗传算法求函数最小值的Python示例代码:
```python
import random
# 定义目标函数
def fitness_func(x):
return x ** 2
# 初始化种群
def init_population(pop_size, chromo_len):
return [[random.randint(0, 1) for _ in range(chromo_len)] for _ in range(pop_size)]
# 计算个体适应度
def calc_fitness(population):
return [fitness_func(int(''.join(str(gene) for gene in individual)), 2) for individual in population]
# 精英选择
def select_elite(population, fitness):
elite_idx = fitness.index(min(fitness))
return population[elite_idx]
# 交叉操作
def crossover(parent1, parent2):
crossover_point = random.randint(0, len(parent1) - 1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
# 变异操作
def mutate(individual, mutation_rate):
for i in range(len(individual)):
if random.random() < mutation_rate:
individual[i] = 1 - individual[i] # 二进制基因取反
return individual
# 遗传算法主函数
def genetic_algorithm(pop_size, chromo_len, max_generations, mutation_rate):
population = init_population(pop_size, chromo_len)
for _ in range(max_generations):
fitness = calc_fitness(population)
elite = select_elite(population, fitness)
new_population = [elite]
while len(new_population) < pop_size:
parent1 = random.choice(population)
parent2 = random.choice(population)
child1, child2 = crossover(parent1, parent2)
child1 = mutate(child1, mutation_rate)
child2 = mutate(child2, mutation_rate)
new_population.extend([child1, child2])
population = new_population
best_solution = select_elite(population, calc_fitness(population))
return int(''.join(str(gene) for gene in best_solution), 2)
# 示例调用
pop_size = 100 # 种群大小
chromo_len = 10 # 染色体长度
max_generations = 50 # 最大迭代次数
mutation_rate = 0.01 # 变异率
best_solution = genetic_algorithm(pop_size, chromo_len, max_generations, mutation_rate)
print("最小值为:", best_solution)
```
在示例代码中,我们定义了一个简单的目标函数 `fitness_func(x)`,并使用二进制编码来表示染色体。通过选择、交叉和变异等操作,不断优化种群中的个体,最终得到一个逼近函数最小值的解。通过调整种群大小、染色体长度、迭代次数和变异率等参数,可以对算法的性能进行调优。
相关推荐















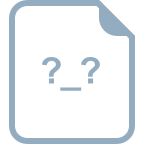
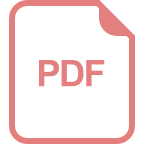