遗传算法求解函数最小值的python实现
时间: 2024-10-12 08:00:36 浏览: 21
遗传算法是一种模拟自然选择和遗传机制的优化搜索技术,用于解决复杂优化问题。在Python中,我们可以使用`deap`库来实现遗传算法,它提供了一个高级API来处理这个问题。
以下是一个简单的遗传算法求解函数最小值的Python示例,假设我们有一个需要最小化的函数`f(x)`:
```python
import random
from deap import base, creator, tools
# 定义问题函数和适应度函数
def fitness_function(solution):
# 这里替换为你要优化的实际函数,比如 f = x**2 + y**2
return - solution[1]**2 # 假设我们要最小化x和y的平方和
# 创建遗传算法的环境
creator.create("FitnessMin", base.Fitness, weights=(-1.0,))
creator.create("Individual", list, fitness=creator.FitnessMin)
toolbox = base.Toolbox()
toolbox.register("attr_float", random.uniform, -10.0, 10.0)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_float, n=2)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
toolbox.register("evaluate", fitness_function)
toolbox.register("mate", tools.cxTwoPoint) # 随机交叉操作
toolbox.register("mutate", tools.mutGaussian, mu=0, sigma=1, indpb=0.1) # 高斯变异
toolbox.register("select", tools.selTournament, tournsize=3) # 择优竞赛
def main(population_size, generations):
pop = toolbox.population(n=population_size)
hof = tools.HallOfFame(1) # 保存最佳解决方案
for gen in range(generations):
offspring = toolbox.select(pop, len(pop))
offspring = [toolbox.clone(ind) for ind in offspring]
# 交叉和变异
for child1, child2 in zip(offspring[::2], offspring[1::2]):
if random.random() < 0.5:
toolbox.mate(child1, child2)
del child1.fitness.values
del child2.fitness.values
for mutant in offspring:
if random.random() < 0.2:
toolbox.mutate(mutant)
del mutant.fitness.values
# 评估新个体的适应度
invalid_ind = [ind for ind in offspring if not ind.fitness.valid]
fitnesses = toolbox.map(toolbox.evaluate, invalid_ind)
for ind, fit in zip(invalid_ind, fitnesses):
ind.fitness.values = fit
pop[:] = offspring
# 保存当前最佳解
best_ind = tools.selBest(pop, 1)[0]
hof.update([best_ind])
return hof[0] # 返回最终找到的最佳解
# 调用主函数并设置参数
best_solution = main(population_size=100, generations=100)
print(f"最优解:{best_solution} (fitness={fitness_function(best_solution)})")
阅读全文
相关推荐
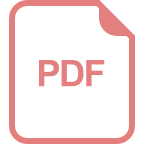
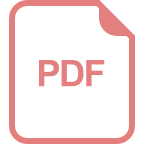



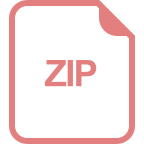




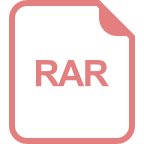
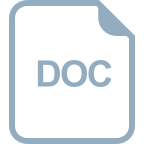
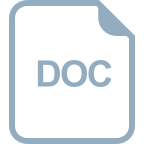


