python遗传算法求函数最小值
时间: 2024-06-13 07:02:53 浏览: 18
Python中的遗传算法是一种模拟自然选择和遗传机制的优化算法,用于解决复杂问题,如寻找函数的最小值或最优解。遗传算法主要包含以下几个步骤:
1. 初始化种群:随机生成一组解(称为个体或染色体),这些解代表可能的函数值。
2. 适应度评估:计算每个个体对应的目标函数值,适应度越高,表示该个体越接近解。
3. 选择:基于适应度值,选择一部分个体进入下代,概率较高的个体被选中的可能性越大。
4. 变异:对选择的个体进行变异操作,可能包括基因突变(改变某个基因)或交叉(交换部分基因)。
5. 进化:重复步骤3和4,逐步迭代种群,直到满足停止条件(如达到预设的迭代次数或适应度达到预设阈值)。
6. 返回最优解:最终的种群中,适应度最高的个体通常就是所求的函数最小值近似解。
在Python中,你可以使用一些库如`deap`(Distributed Evolutionary Algorithms in Python)来实现遗传算法。以下是一个简单的例子:
```python
from deap import base, creator, tools
# 创建适应度函数和种群
creator.create("FitnessMin", base.Fitness, weights=(-1.0,))
creator.create("Individual", list, fitness=creator.FitnessMin)
def evalOneMax(individual):
return -sum(individual), # 函数最小值对应最大适应度
toolbox = base.Toolbox()
toolbox.register("attr_bool", random.randint, 0, 1) # 二进制编码
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_bool, n=10)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
toolbox.register("evaluate", evalOneMax)
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutFlipBit, indpb=0.05)
toolbox.register("select", tools.selTournament, tournsize=3)
# 运行遗传算法
pop = toolbox.population(n=50)
best_gen = tools.selBest(pop, k=1)
for gen in range(100): # 迭代次数
offspring = toolbox.select(pop, len(pop))
offspring = [toolbox.clone(ind) for ind in offspring]
for child1, child2 in zip(offspring[::2], offspring[1::2]):
if random.random() < 0.5:
toolbox.mate(child1, child2)
del child1.fitness.values
del child2.fitness.values
for mutant in offspring:
if random.random() < 0.2:
toolbox.mutate(mutant)
del mutant.fitness.values
invalid_ind = [ind for ind in offspring if not ind.fitness.valid]
fitnesses = toolbox.map(toolbox.evaluate, invalid_ind)
for ind, fit in zip(invalid_ind, fitnesses):
ind.fitness.values = fit
pop[:] = offspring
best = tools.selBest(pop, k=1) # 获取当前最佳解
print("Best individual: ", best, " with fitness: ", best.fitness.values)
```
相关推荐
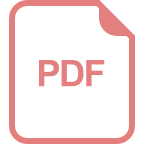














