如何编写一个程序,以便从输入的字符串中移除特定的字符,并区分大小写不同的相同字母?
时间: 2024-12-26 10:28:04 浏览: 3
要编写一个程序来从输入的字符串中移除特定的字符并区分大小写,你可以采用以下步骤:
1. **接收用户输入**:
首先,你需要创建一个函数或方法,让用户输入他们想要移除的字符以及原始字符串。
```python
def remove_char(input_string, char_to_remove):
```
2. **遍历字符串**:
使用循环结构(如for或while),遍历输入字符串的每个字符。对于Python,可以使用`str.replace()`函数,但如果需要区分大小写,就直接比较字符。
```python
for char in input_string:
if char.lower() == char_to_remove.lower(): # 比较小写版本,区分大小写
input_string = input_string.replace(char, '', 1) # 用空替换字符
```
3. **返回结果**:
循环结束后,返回处理后的字符串。
```python
return input_string
```
4. **完整代码示例**(Python):
```python
def remove_char(input_string, char_to_remove):
result = ""
for char in input_string:
if char.lower() != char_to_remove.lower():
result += char
return result
# 测试示例
input_str = "Hello, World!"
char_to_remove = 'o'
output_str = remove_char(input_str, char_to_remove)
print(f"原字符串: {input_str}")
print(f"移除 '{char_to_remove}' 后: {output_str}")
```
阅读全文
相关推荐
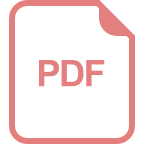
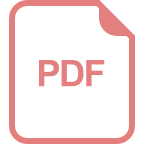
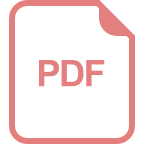















