在arduino中,利用温度传感器1个,电位器一个,按钮1个,LCD显示屏1个,舵机1个。要求旋转电位器在180°范围内使舵机转动同时在LCD上显示温度,松开按钮为摄氏温度,按下按钮为华氏温度,写出代码
时间: 2024-10-18 16:07:07 浏览: 61
在Arduino中,实现这个功能需要编写基本的硬件初始化、输入读取以及温度转换的代码。这里是一个简单的示例,假设我们使用了DS18B20温度传感器、Potentiometer(电位器)、Button(按钮)、LCD1602液晶屏以及servo库控制舵机:
```cpp
#include <Servo.h>
#include <LiquidCrystal.h>
#include "OneWire.h"
#include "DallasTemperature.h"
// 定义硬件连接
const int potPin = A0; // 电位器连接到A0
const int buttonPin = 2;
const int servoPin = 9;
LiquidCrystal lcd(12, 11, 5, 4, 3, 2); // LCD连接
Servo myservo;
OneWire oneWire(Wire);
DallasTemperature sensors(&oneWire);
void setup() {
Serial.begin(9600); // 开启串口调试
pinMode(potPin, INPUT);
pinMode(buttonPin, INPUT_PULLUP);
lcd.begin(16, 2); // 初始化LCD
myservo.attach(servoPin);
}
void loop() {
// 读取电位器值并计算舵机角度
int potValue = analogRead(potPin);
int angle = map(potValue, 0, 1023, 0, 180); // 0-1023对应0-180度
// 更新舵机角度
myservo.write(angle);
// 温度测量和显示
if (digitalRead(buttonPin) == LOW) { // 按钮未按下(低电平)
sensors.requestTemperatures(); // 请求温度读取
float tempC = sensors.getTempCByIndex(0); // 获取第一个传感器温度(通常是DS18B20的温度)
lcd.setCursor(0, 0);
lcd.print("Temperature:");
lcd.print(tempC, 1);
lcd.setCursor(0, 1);
lcd.print("°C");
} else { // 按钮按下(高电平)
lcd.clear();
float tempF = celsiusToFahrenheit(tempC); // 转换为华氏温度
lcd.print("Temperature:");
lcd.print(tempF, 1);
lcd.setCursor(0, 1);
lcd.print("°F");
}
delay(1000); // 1秒刷新一次
}
float celsiusToFahrenheit(float celsius) {
return (celsius * 9.0 / 5.0) + 32; // 华氏温度公式
}
```
请注意,这个代码片段是一个基础示例,实际应用中可能需要添加错误处理和延时等细节。此外,DS18B20的初始化部分需要根据具体硬件和库进行适当的配置。
阅读全文
相关推荐
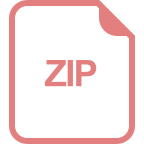
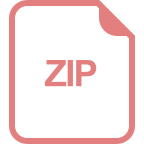
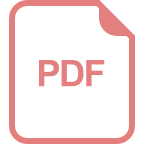






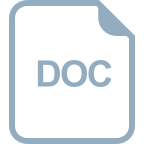
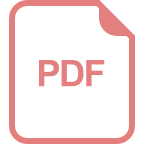
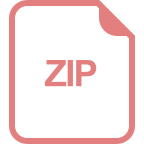
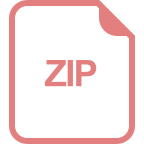
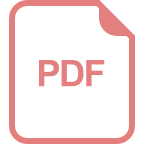